pico-view:
December 2023
Hello Pico-View Reader, this December issue is the twelfth in the series. 12 issues in 12 months for the entire 2023 year! Because of that, this issue will be a little different, as we don't just look back on the month of December, but the entire year! What a year it has been!
To truly mark this special occassion, this incredible PETSCII style animated cover art was created by the one and only, creator of PICO-8, Zep! What an honor, thank you!
And as always, thank you to the writers, contributors, and all supporters, that includes you reader! We thank you from the bottom of our hearts. And with that, have fun adventuring through the pixels and paragraphs of this issue of the Pico-View web-zine!
Authors:
Tubeman, Lokistriker, LouieChapm, Johan Peitz, SaKo, Kuma Khan22, Achie, and Nerdy Teachers
Special Thanks to Our Supporters:
Xrqton, Johan Peitz, user1366, Tréveron, discorectangle, dcolson, bikibird, Noh, RidgeK, RotundBun, sourencho, Yoko, Traxxasislife, Tubman, psicolabile
Contents:
-Cover Art by Zep
-Playing with Visual Effects - Tubeman
-December Games Rapid-fire Reviews - Achie
-Top 10 Games of 2023 - NerdyTeachers
-Developer Interview - LouieChapm
-Developer Interview - LokiStriker
-Apskeppet: Year in Review - Johan Peitz
-Curated Games Library - NerdyTeachers
-Outstanding Outliers - KumaKhan22
-Pixel Art Gallery and Interview - SaKo
----------------------------------------------
-The Past and Future of Pico-View - Nerdy Teachers
-Closing Remarks
Playing with Visual Effects
by Tubeman
Inspired by watching demoscene productions in PICO-8, I've recently fallen in love with making small throwaway visual effects (basically tweetcarts without the 280 character limit). This has led me down a path of learning things like manipulating sine waves and basic 3D rendering. Here, I'll walk through the process of creating a basic effect (including some of the parts that I end up undoing).
Making a Polygon
Let's start by drawing a polygon, with an adjustable number of vertices.
ngon=3 -- number of polygon vertices
function _update()
if (btnp(⬅️)) ngon-=1
if (btnp(➡️)) ngon+=1
pts={}
for a=-1,1,1/ngon do
add(pts,{
x=64+32*cos(a),
y=64+32*sin(a),
})
end
end
function _draw()
cls()
for i=1,#pts do
local p1,p2=pts[i],pts[i+1]
-- connect last & first point
if (i==#pts) p2=pts[1]
line(p1.x, p1.y, p2.x, p2.y, 7)
end
color() cursor()
print("ngon "..ngon)
end
Note that we recreate the pts
array every _update
in order to easily configure things at runtime such as the number of points.
Animating the Polygon
To rotate the polygon, we can add a phase shift to the x/y calculations by changing e.g. cos(a)
to cos(a+t()/8)
. Note that we divide time by 8 to slow down the rotation. However, it might be handy to play with the speed of the rotation, so let's make it adjustable:
rotspd=1/8 -- rotation speed
function _update()
if (btnp(⬆️)) rotspd+=.1
if (btnp(⬇️)) rotspd-=.1
-- ...
add(pts,{
x=64+32*cos(a+t()*rotspd),
y=64+32*sin(a+t()*rotspd),
})
end
function _draw()
-- ...
print("rotspd "..rotspd)
end
We can also animate the radius of the polygon by replacing the amplitude with a value that oscillates between 16 and 48:
-- linear interpolation
-- eg. `lerp({10,20}, .5)` returns 15
function lerp(ab,t) return ab[1] + (ab[2]-ab[1])*t end
-- map a value that ranges from [-1,1] to the given interval
-- eg. use `map(cos(t()), {-3,6})` to oscillate between -3 and 6
function map(v,ab) return lerp(ab, v/2+.5) end
function _update()
-- ...
for a=-1,1,1/ngon do
rad=map(cos(t()/2), {16,48})
add(pts,{
x=64+rad*cos(a+t()*rotspd),
y=64+rad*sin(a+t()*rotspd),
})
end
end
Adding color
Next, we can animate the color using the bright colors between 8-16:
f=0 -- frame count
function _draw()
f+=1
-- ...
line(p1.x, p1.y, p2.x, p2.y, f%8+8)
end
I noticed that removing the cls()
(and adding it to button handlers) created a neat effect:
This made me want to try adding screen decay in lieu of cls()
:
cls()
function _draw()
for _=1,3000 do
local a=0x6000+rnd(0x2000)\1
poke(a, peek(a)*.01)
end
-- ...
end
Note that the decay interferes with the debug text, so let's give the text a black background using the "\#0" P8SCII control code:
print("\#0ngon "..ngon)
print("\#0rotspd "..rotspd)
We now have something like this:
I played around some more with the rotation and radius and found something neat, but decided to scrap it:
Playing with thickness
Next, I played around with the thickness of the polygon lines. To do this, I took the simplest approach of drawing additional lines with an offset:
thick=3 -- polygon thickness
function _update()
if (btnp(🅾️)) thick-=1
if (btnp(❎)) thick+=1
-- ...
end
function _draw()
-- ...
for ox=0,thick-1 do
for oy=0,thick-1 do
line(p1.x+ox, p1.y+oy, p2.x+ox, p2.y+oy, f%8+8)
end
end
-- ...
print("\#0thick "..thick)
end
This gave a neat effect, but a bit too intense with the color strobing, so I tried some fill patterns:
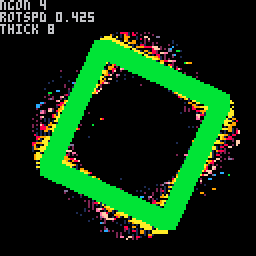
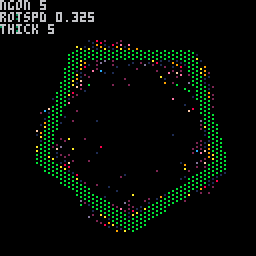
Adding Concentric Polygons
After some thought about where to go next, I decided to scrap the line thickness idea and replace it with concentric polygons. The easiest way to achieve this is to move the 64+rad*...
from _update
into _draw
:
function _update()
-- ...
add(pts,{
x=cos(a+t()*rotspd),
y=sin(a+t()*rotspd),
})
end
function _draw()
-- ...
for r=60,4,-8 do
for i=1,#pts do
local p1,p2=pts[i],pts[i+1]
-- connect last & first point
if (i==#pts) p2=pts[1]
line(64+r*p1.x, 64+r*p1.y, 64+r*p2.x, 64+r*p2.y, f%8+8)
end
end
end
Here's the result:
Adding Oscillators
Our polygons feel a bit stiff, so let's try making some of the static values dynamic. We can do this using oscillating values. Let's start with the radius of the largest polygon. Similar to the rotation speed, we make the radius speed adjustable:
-- like `map` but takes a value between [0,1]
function map2(v,ab) return lerp(ab, v) end
radspd=1/3 -- radius speed
function _draw()
-- ...
rad=map2(cos(t()*radspd+.5)^.5, {6,60})
for r=rad,4,-8 do
-- ...
end
Now the radius animates between 6 and 60. We use a square root in order to change the cos
wave into something more burst-y.
Next, let's make the rotation oscillate instead of turning in a single direction.
rotspd=1/2
radspd=1/3
function _udpate()
-- ...
rot=map(cos(t()*radspd+.5), {-.4,.4})
add(pts,{
x=cos(a+rot*rotspd),
y=sin(a+rot*rotspd),
})
end
Note how we use radspd
to make the rotation's frequency matches the radius's frequency. This makes the rotation increase while the radius is increasing, and decrease while the radius is decreasing:
Also note that we offset the phase by adding 0.5 in order to make the rotation start at beginning of the interval (cos(.5)
is -1).
Next, let's make the gap between polygons dynamic by oscillating the for-loop's step:
function _draw()
-- ...
step=map(cos(t()*rotspd+.5), {8,40})
for r=rad,4,-step do
-- ...
end
Note that we make the amplitude based on the rotation speed (instead of radius speed) in order to make the gap animation a bit incrongruent with the radius animation.
Lastly, let's tweak the colors
function _draw()
-- ...
ri=16
for r=rad,4,-step do
ri-=1
-- ...
line(64+r*p1.x, 64+r*p1.y, 64+r*p2.x, 64+r*p2.y, ri-f%5)
end
This makes each polygon's color a function of both time ( f
) and its index ( ri
). Here's the final result:
Going Further
There are many tools and techniques in PICO-8 to add to your toolbelt, including 3D rendering, tline()
, map()
, bitplanes, making a triangle rasterizer, etc. There are also countless old-school demoscene effects to try to recreate (e.g. plasma, twisters, rasterbars) as well as mathematical phenomena to explore (e.g. chaos games, fractal trees, boids).
If you enjoy PICO-8 and making visually pleasing things, you should give this a try!
December Games
Rapidfire Reviews
by Achie
Finding a game to review each month is not that easy, for me at least. Sometimes of course one of your favorite devs drops a game and you got the jackpot, sit back, relax you know what you want. On the other hand though, that doesn't happen always, and now you're gonna have to play all these cool little games people make, and find only ONE that you think is fire enough to deserve a spotlight. Who am I to choose that? And this was one of those months.
Moreover I'm a selfish man, who wanted to have 12 reviews for 12 Pico-View issues, cuz you know, that sounds nice. That would mean I'd have to review 2 because I missed February, which is cool in the end because that started the whole "Random Review" section.
So here I am, sitting in front of the computer, Word open, BBS pages turned back and forth, trying to find 2, and then it hit me. What if I finish the year with a bang! Hijack Random Reviews and do a Rapid-fire Review for the seasons with like 10+ games?! Nerdy cannot stop me, he is not gonna see it until I submit, so what can he do about it? NOTHING *insert evil laugh*
( Editor's Note: Yes...this is accurate... )
So anyway, Ultra-Epic-Super-Mega-Review-Bonanza let's goooooo!
Medusa: Aegis of Life
by Chico
Medusa: Aegis of Life is a cool little starting stage of a nice mythology based score chaser! Help Medusa the gorgon to petrify the heroes trying to hunt her down in her little cave! Movement and timing is crucial for survival, can’t wait to see it expanded!
Grav
by Noh
Grav grabs the usual fast paced nature of Noh’s game library and puts you into a gravity flipping platformer speedrun course to test your reflexes, memory, and platforming skills! Backed by PicoTunes the game unleashes ultra speed, which you need to tame to get to the end!
Phive Nights At Pico’s
by DragonXVI
A perfect remake of the original games, which I haven’t played, so who is the remake now? Sit in the dark security room and watch the cameras as the place is more alive than you would think! Nice sounds and visual designs, an amazing work overall! Try it out yourself for some end of year frightening!
Pizza Panic!
by CodeNameWaddles
Put on Deja Vu or Tokyo Drift and enjoy the life of a mad pizza delivery guy drifting and colliding through the city trying to deliver as much pizza as humanly possible. If only DoorDash would be this cooperating! Lovely top-down graphics, physics jank enough and a bopper own music track, worthy of drifting Mozzarella!
Looping Larceny
by bluswimmer and AbyssalMari
What happens when you combine a metroidvania with minit-s cyclic exploration? This is what they asked themself while making Looping Larceny an amazing little exploration game where time is crucial! Explore this cute little world, find the upgrades and acquire that precious hidden diamond the rumors are about!
Badland Caravan
by DieterHorvat
Delve into the arcade cabinet caves and use your sword to defend yourself in this survival arcade score chaser! Will the spiders overwhelm you, or will you be able to use the pickups to your advantage? Cute stylized graphics, a banger soundtrack and many many spiders await you! So what are you waiting for? Insert that coin!
Mono Beam
by PeterHodgden
I’M FIRING MY LASOOOOOR! Jokes aside, you are firing a single laser beam to toggle switches, bounce off mirrors and other beams to solve the puzzle of each room! All this in a cute graphical and sound package makes an amazing little game to bash your head into the desk with! Can you solve each room? Or will your ideas bounce off the mirrors?
Socks
by voidgazerBon
Pairing socks to hang them out to dry is boring … but have you tried gamifying it? voidgazerBon did exactly this as we follow Billy and the endless pair of socks to pair. Drying, falling, exploding, moved by the wind next time you tell me they are gonna freeze … sheesh. Follow the journey through the year of chores and then realize you actually enjoy pairing socks. Preposterous.
Holiday Bundle
by TheTomster
Is it cheating to review a bundle? You know what, I don’t care, this is already written by the time you answer it! Tom Hall again surprised us with gathering amazing people from the community to publish an amazing bundle of holiday themed games! Jump into and enjoy the endless Christmas fun! 9 games inside for you to try and hey, maybe next year you will join them!
Gravico
by hiyaa
Remember Dr. Mario? hiyaa made an amazing twist on the falling block and color matching genre with one simple change. Blocks only stay in place, if something is under them. Explore the options and puzzles of a gravity based matching game! Build smartly and abuse chaining combos for more and more points! Amazing entry for a first game in a clean and tight package, well done!
Chimney
-by masa_rst
Masa_rst grabbed the idea of Christmas and threw it down a chimney, quite literally! Help Santa descend the variously shaped chimneys in this level/score chaser! May the festivities be with you to help avoid the sometimes questionable brickwork. At least they are clear of soot!
Pilot Kim
-by Randsak
Following the works of the LazyDevs shmup tutorial Randsak created a small little dynamic wave based shmup, where you can select random upgrades after each stage and earn new weapons along the way! Waiiiiit … is this a roguelike? Reeee! Cute little graphics, nice alien designs, overall really well made! 10 stages is all you have to beat!
Cyber Santa
-by kadoyan
Ever wondered what would happen if Santa would spread gifts in a cyberpunk world? With a powerful Sandevistan attached to him? Slow down time, avoid bullets and spread your gifts before the bullets and the hackers get to you in this bullet-hell shoot-em-up dodge score chaser game! Amazing graphics, sound effects, and a banger track awaits you in the future. Are you Santa enough? Let’s see.
Amidar
-by pahammond
Jump back in time into the Konami era with this remake from 1981! Jump into the role of a coconut gathering gorilla or a stage painting paint roller, but one thing is always there, the Amidar! Avoid them at all cost, while remembering or (as me) first playing this amazing remake in the PICO-8 library!
About the Author
I stream PICO-8 gameplay and PICO-8 game development on Twitch, as well as write detailed dev logs and a game review series called "Pico Shorts".
Thanks for reading!
Top 10 PICO-8 Games of 2023
(Based on BBS Stars)
(Based on BBS Stars)
by NerdyTeachers
Thanks to running Pico-View all year, our team has had eyes on the BBS like never before, playing as many games as we could to catch all the note-worthy releases each month and sometimes sit down with the developers to work on articles together or give an interview.
One remarkable consequence of that is the ability to gather enough data from the BBS to be able to make a list of 2023 PICO-8 releases and order them by the number of Star Ratings they received. Without doing a whole separate nominations and voting system for the best games, this gives us the next closest community ranked PICO-8 game awards for this year!
So without further ado, here are the results (plus 2 more):
(Star Rating data updated on 28 December 2023)
Congratulations to all of these developers! I hope you can all look back at these amazing games you've created this year with pride in knowing how much they have been and still are being enjoyed.
One thing we have noticed after collecting so much BBS data is the unfortunate trend in giving stars has fallen dramatically over the years. There are a lot of factors that can influence that, one possibly being that we don't see the influence that it has on a game (I know I'm guilty of this). So our hope is to bring more community attention back to the star ratings, showing you that your stars do matter. So the next game you play on the BBS that you enjoy, don't forget to take the extra few seconds to give it a star, and let the developer know by leaving a comment. You can even start with these top rated games of 2023, congratulating the devs!
We reached out to the developers of the top 2 games and broke the good news early, congratulating them directly on this achievement, and asked if we could have extended interviews with them specifically about these two PICO-8 masterpieces.
So prepare yourselves with a nice hot beverage and get comfy to fully enjoy these Developer Interviews, up next.
Developer Interview
with LouieChapm
Nerdy: Hey Louie, how's it going?
Louie: I'm going good! Still working on secret Kalikan stuff, motivation is high. Basically as good as where I'd like to be!
Nerdy: You're no stranger to Pico-View as you wrote a great article in our very first zine way back in January, then later your game Calico received a Featured Review, and we were both judges in the Cre8 Jam. It's been great chatting and getting to know you this year so I'm really pleased to be able to come full circle and talk to you about your PICO-8 games and achievements in our final zine of the year.
Louie: As always, it's lovely to talk!
Nerdy: Possibly one of your greatest achievements this year was the game Kalikan! For anyone who hasn't played it yet, how would you describe the game?
Louie: Kalikan is a "danmaku" or "bullet curtain" shoot 'em up (shmup), which is a subgenre of shooting type games that feature walls (or curtains?) of projectiles being fired at you, and are normally fairly difficult! From the get-go, my goal with Kalikan was not to reinvent the genre—but instead to celebrate the simple mechanics that makes it so enjoyable.
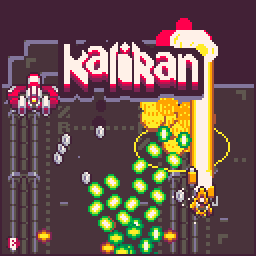
Nerdy: How did it feel to learn that Kalikan was given the most stars on the BBS this year and therefore essentially voted as the #1 PICO-8 game of 2023?
Louie: Oh my, it was lovely. I really wasn't expecting the response at all. I've always just made games for me and my friends, and Kalikan made me realise I've got far more friends than I thought! The visibility also allowed me to connect with so many inspiring and talented people. It was really nice to have such a vast pool of knowledge to draw from, and honestly- is the only reason why I was able to put so much polish on the game. It really feels like a group project that I just happen to direct. It's been so nice :')
Nerdy: It's awesome to be able to draw from and work with the community like that. Can you share your inspiration behind the visual design of the game? The sprites, color palette, and backgrounds in Kalikan are absolutely gorgeous.
Louie: I was so flattered that the visuals became such a distinguishing feature, because I don't really consider myself that confident a pixel artist. The influence of Dodonpachi is pretty hard to miss, from enemies to UI my love for that game is pretty apparent. Krystian, Jammigans, Lokistriker, Alethium, and Ericb were all all SUPER instrumental to the visual language of Kalikan, each in their own way- and I love seeing anything they all produce.
Nerdy: When you reach the first boss and that giant red moon appears, it's such an epic moment to give the players a sense of awe and reward for reaching it. I'm curious if that was that an early design decision or if that came later in the process. How did you approach creating those visually immersive moments in the game?
Louie: The giant evil moon, I'd say, is the most iconic piece of visual design in Kalikan —programmed pretty much entirety by @nyeogmi it served as such a great visual landmark to anchor everything against. Funnily enough, the moon was actually one of the first things that was really cemented into the game! I think Nyeogmi and I were really on the same page, they are unbelievably clever.
Nerdy: Nyeogmi is great, they are really active and helpful and in the PICO-8 Discord. To have an anchor like that to ground and tie the rest of the game's aesthetic to is really great to have early on!
Louie: As for creating exciting moments, I drew a lot of inspiration from GG Aleste 3. Krystian does a fantastic job of explaining my mindset in his video "The Toybox Storytelling of GG Aleste 3" which does such a great job of putting to words what I felt about shmup environment design. I highly recommend giving it a watch.
Nerdy: Speaking of Krystian from Lazy Devs Academy (LDA), he has been pumping out fantastic shmup tutorials. However that series is not yet complete and you released Kalikan before most of that series even came out. So was Kalikan born from and influenced by those tutorials and if so, at what point did it take off on its own?
Louie: What an excellent question. Kalikan exists solely because of those tutorials! LDA's beginner shmup tutorial actually kickstarted my interest in shmups, as I'd never actually played one before. Having a tutorial to lean against, as well as Krystian simplifying and breaking down the genre, was really the basis of my entire understanding of shmups. Even beyond that too, a lot of the systems are just my interpretations of the systems he has designed- the modular approach to a projectile spawner was entirely his invention!
My first shmup Warwind which was my submission for LDAs Basic Shmup Showcase is a really interesting comparison too. It was thought of fairly positively by non-shmup players, but loathed by people familiar with the genre. When Krystian announced his Advanced Shmup Tutorial series I wanted to make a shmup that shmup players can really enjoy; And thus, Kalikan was born!
Another huge influence was Aktane's CrossGunr, prior to that I didn't think that shmups were actually possible in PICO-8. I always considered the screen to be too small for it to really work. Which is funny in retrospect, because we've got so many incredible shmups now! xD
Nerdy: It's funny you say that about Warwind because I must admit, as a gamer, I'm not a fan of shmups because they can quickly become overwhelming with visual clutter and sudden jumps in difficulty. But I actually love Kalikan and I return to play it often!
Louie: It's a funny thing, because I totally agree —I also find most shmups really overwhelming for the same reasons.
I think a lot of people who make shmups, are also competent shmup players. So you end up with a lot of shmups that are made for players of a similar skill to the developer- which means they're normally hard as nails. I think being a less confident shmup player made Kalikan feel much more approachable. I had a couple people message me and tell me that they don't normally enjoy shmups, but Kalikan was the first one they loved. Which is such a motivating thing to hear! As well as that, I think being able to talk design with really knowledgeable shmup developers, and having constant playtesting/advice was so handy too. Being guided through not only what the genre standards are, but also why they are standards too was super handy!
Nerdy: That's a great tip for game devs; know your own skill level and seek playtesters who are at varying skill levels from yourself to be able to tailor your game to a wider audience of players.
During development of Kalikan, you showcased impressive technical aspects, especially with the custom editors you made. Can you highlight any innovative or challenging technical achievements you're particularly proud of?
Louie: So many of my favourite things about Kalikan were coded alongside other people. Naturally Nyeogmi's moon is something I'm so proud to have in my game, I love it so much that it's front and centre on the store page!
Beyond that, I'm really proud of my bullet system. Originally based on LDA's bullet system, there's a couple features in there unique to Kalikan, primarily circ
and filters
.
circ
basically allows you to define a bullet to spawn in a circle shape with an arbitrary radius and bullet count. Really good for creating nasty patterns that you have to macrododge!
filters
are a bit harder to explain, so I won't get too technical, but because the bullets have no reference to the object that spawned them after they exist, filters are a way to give extra commands to projectiles after they have spawned. They allow me to slow down / speed up shots that are already alive; change their direction; or even spawn entirely new patterns at a fixed rate. There's so much depth in this system that I'm still figuring tricks out myself! xD
Nerdy: The artist in me is mesmerized by the bullet patterns, and the gamer in me wants to get in there and figure out how to dodge them ?.
Bullet pattern variety is integral to an interesting shmup, and the same goes for variety of player and enemy ships. I remember shortly after releasing Kalikan with the original yellow ship, you added the second ship with its own movement and special abilities. But you've teased us with a third ship that is still locked. What are your plans for that?
Louie: Ah, so... I may have backtracked a little on the variety in the ships in Kalikan. As of right now the differences between the ships has been simplified greatly. I played around with some other ideas, but honestly I always just keep coming back to the Type B with the laser. The variety is fun, but I'd rather have a balanced experience for the superplayers who are interested enough to learn the game. There'll definitely be a third ship in Kalikan, but as far as gameplay- you might have to wait and see. ;)
My dream is that the top 10 scores are equally divided across the three playable ships.
Nerdy: Love that, and I think that's a great goal to have while balancing the ships. Well I for one will be impatiently waiting for that third ship?. Especially because I know you have Kalikan 2 in development and we are very excited for it! How does this fit in with the original Kalikan? Is it an extension with continued levels, or a separate game entirely, more like a sequel?
Louie: You know, at this stage I'm not quite sure. My current thinking is that it's a continuation of the original, but with big enough changes to be its own separate thing. Kalikan as it exists online feels like more of a tech demo, or a teaser- to the actual thing. The moon / shuttle level will still feature, but with completely changed enemies and patterns to avoid. >:)
Nerdy: Oh wow, that's even more exciting to think that Kalikan was just an appetizer for what you have in store! When do you think we can expect to play it and are you looking for playtesters??
Louie: It's so far away from being finished I can't really even give an estimate for a release, to be honest. Level design takes a long time, and I'm doing it mostly on my own- so it's a slow process. I'll probably get some help later down the line, but at this stage I've got no idea when it'll be finished. Regarding playtesters, I'm always looking for help with level design. I'm fairly new to this genre, so feedback is always appreciated.
Nerdy: Totally understand about the level design process. Before adjusting the level to playtesting feedback, what is your approach to the initial designs?
Louie: My approach is fairly iterative, pretty much everything faces several major changes from the first moment it's in game. I'm still learning so much, so my approach changes pretty radically.
Even just going back and playing through the original Kalikan, I can see how much better at level design I am now. Hopefully, I'll look back at my current work and feel the same way- although, I'd love if I didn't need to rework it all again. xD
Nerdy: Could we get a sneak peek at a level you're working on?
Louie: Of course!
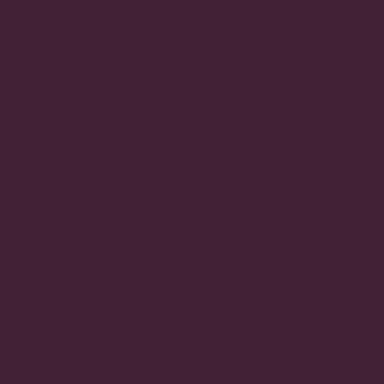
Nerdy: That looks amazing, with some familiar enemies and a few new ones in there too. I think everyone will be really happy to know that we still have a lot to look forward to with Kalikan. I'm sure that Kalikan will inspire more high-polished and well-balanced shmups next year. What advice would you give to those aspiring game developers, especially ones looking to create an all around great gaming experience in PICO-8?
Louie: Thank you :^) It's been a blast designing enemies for Kalikan! I'd be honoured if Kalikan inspired more shmups, and it looks like there's already a lot to look forward to too!
As for advice, reach out to people that inspire you. I've had so many conversations with incredibly folk over the course of Kalikan, and its made it better tenfold. Plus, the PICO-8 community has got to be one of the friendliest circles on the internet! xDD
Nerdy: I definitely agree! Thanks for sitting down with us for this interview and sharing your time and insights into your game development methods. And once more, congratulations on making the #1 PICO-8 game of the year! We are definitely looking forward to seeing what you do with 2024.
Louie: Always a pleasure Nerdy! I hope you're able to gleam something interesting from all this!
And thank you! For a year filled with such great releases, its such an honour to be there. I'm really on the shoulders of giants here.
Nerdy: It's been truly great, well hope you enjoy the holidays and have a happy new year!
Louie: You too <3
Developer Interview
with LokiStriker
Nerdy: Loki, good morning, how are you?
Loki: Been doing good! Thank you for asking. Getting ready for the incoming holidays.
Nerdy: Good ol' December, holiday season, where we look back on the entire year and welcome in the next. This past year has been great working with you multiple times on Pico-View articles where you've discussed a wide variety of topics from music to token saving! And this is actually your second interview with us as well, so welcome back!
Loki: Well, thank you for having me back! Its always a pleasure to write for the zine.
Nerdy: I don't know if you know this, but the first game I put on my dedicated PICO-8 arcade was Beckon the Hellspawn (BTH), and as I browse my collection, I am still always reaching for it after countless plays.
Loki: Oh wow! That means a lot! I'm glad you like it as much as you do. The reception of the game was mostly positive and it was certainly the first game that I really felt proud of.
Nerdy: I really do, and I recommend it often to others but I think describing a game of this genre is still new and tough with terms like Survivors-like, Horde Defense, and Bullet Heaven. How would you best describe Beckon the Hellspawn?
Loki: I personally call it Survivors-like, just like back in the day (before my time that is) games that were "like the game Rouge" were called "Rogue-like". So, in a way of honouring that tradition, I call it as such. That being said, I do understand the current difficulty with categorizing them, as I've seen "bullet hell" and "Shoot 'em up" tags in some games of this new genre. I think it is part of this new discovery, and in time a new name will stick or we will keep the old one.
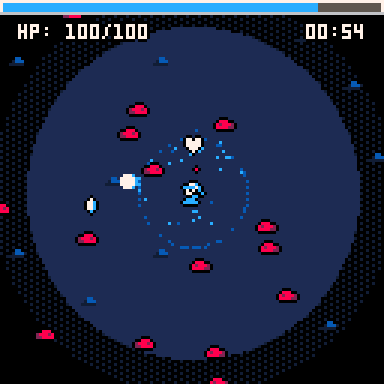
Nerdy: That makes sense to me.
Now BTH was released early in February 2023 and received a rave review in our zine that month. And through the months it has received so many stars on the BBS that it is the #2 PICO-8 game of the year! How does that feel and what were your expectations of the game's reception by players?
Loki: Well, for it being my second game, my expectations of it weren't so high to be honest, and knowing it is the second most rated game of this year is news to me since a couple of days ago. This was not something I was expecting all. Overall, I'm glad the game was found to begin with and that it was well received. I still hold some wishes of how the game could have been better, but I think that overall the game is a good small example of what makes these games so fun to play, which is very gratifying to me.
Nerdy: You initially envisioned a game about a robot in a desert, right? Do you remember what led to the transformation into a wizard and other magical characters battling demons?
Loki: I remember it so well. Too well it fact. This is an aspect of game creation that PICO-8 really made me go through. Sky Fighters, the game released before BTH, was a Space Sim with Combat and Trading with roguelike mechanics. How it ended was an arena topdown shooter. BTH was, yes, a robot fighting in the desert hordes of demons, twin-stick shooter (similar to another Survivors-like called 20 Minutes till Dawn), with some form of meta progression, but that turned into BTH.
The strict limitations of PICO-8, paired with my then current programing skill, demanded of me a more focused design and understand not just the token limits, but my own as well. BTH is really that personal breakthrough of stopping the trend I had of not finishing games, embracing more the idea of compromising but with better, more realistic, design goals.
Nerdy: One of the surprisingly great things about PICO-8, the limitations, but you decided to restrict yourself even more when it came to the palette. With specific color schemes for player and enemy elements. How did you settle on the blue/white for player-related visuals and red/yellow for enemies?
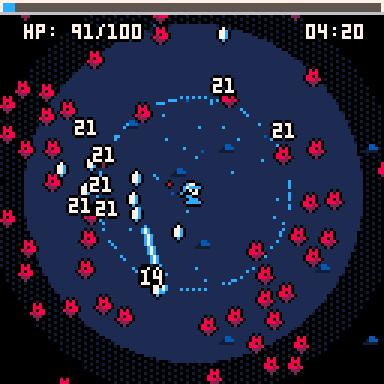
Loki: As a non-(visually)-artistic person, color limitations make it easier for me to develop game assets. Personally, I feel the most comfortable on 1-bit, but since BTH, I've started to feel better with more expanded color schemes.
The contrast of Red and Blue started mostly as the design idea of having a way to easily distinguish what the player should have their eyes drawn towards. This goes for the outlines as well. This idea of having strong colors and outlines paired up with another visual style which was of the NES (Nintendo Entertainment System), 3 colors + transparency for sprites. In my game it was 2 colors for the sprite, black for outline and transparency on the rest. This limitation of the NES informed the rest of the decisions I took with the style.
Nerdy: That's incredible to hear the NES limitations were guiding you here and it certainly worked out really well. And I definitely appreciate the contrast between all the player and enemy sprites on screen.
I've been curious about the playable characters in fact, because they have such interesting names —The Stormcaller, The Moonkin— it invokes the feeling of some unexplained lore in this world you created. Can you share insights into the process of designing these characters and their unique abilities?
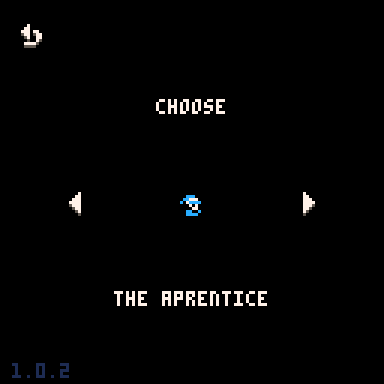
Loki: I truly believe in the power of games (especially old games) to evoke and inspire. In the case of BTH, I wanted to have the characters each be unique, visually and mechanically, and this paired with a name that not simply describes them, but also alludes to deeper context. It gives the player room to see them more fully in their mind than what the graphics can offer.
Mechanically, I wanted to make character selection feel meaningful, and more than just an initial bonus and different looks (like it is for some Vampire Survivors characters), so I tried to make unique variations of each skill, unique to each character, but due to limitations and personally stablished time constraints, I decided to keep the initial characters like the baseline and the last three as the more complex ones. This also gives an easy built in difficulty curve the players can choose to climb. There is some lore in there as well, but I think that if at some point I return to this (let's say) IP, I might delve a little deeper into each, but I still want to keep a sense of wonder and player input in the fantasy of the game.
Nerdy: I love that it was intentional to leave characters vague for players to fill in with their imaginations and I've seen it come through in conversations about which characters different players prefer and it's not just because of their ability differences.
The abilities and the progression system do have a lot of variety to them —that is, they aren't all simply different types of projectiles. How did you decide on which abilities to include and were there any that you tried but eventually didn't make it into the game?
(The Early Days of BTH)
Loki: Well most spells were programmed within the first days of development, so the development goal was mostly getting those into the game, and as time went on, it was clear that adding more was getting near to impossible. I mostly chose them from a selection of spells I liked the most from Vampire Survivors (Garlic, Bible, Ring), while the variations of the spells was more of a tinkering to see what's possible with code. My favorite character is actually the football one (soccer for our USA friends), which is The Possessed, which was actually a random thought turned into one of the most unique gameplays I feel I've done.
Nerdy: "The football one"? I will forever see The Possessed very differently now.
Loki:
(Source code of BTH, proof of the "futbol_blade")
Nerdy: XD That's amazing. I'm always curious what the first major features a developer began with in their game and I wouldn't have guessed it was the spells for you.
Later on though, It must have been difficult when you had to balance the player's attack power (while leveling up) with the enemy's health. Were you number crunching constantly or was that more of an organic adjustment made while playtesting?
Loki: It was mostly a playtesting effort of "feeling" the game rather than "crunching numbers". Since balance is necessary for a game to feel engaging and challenging, I think I made sure to finish the game with every character, but I did balance most combos of spells even before most characters were made, so balance was made with the base spells in mind, rather than the unique ones, because of this, the unique ones tend to have higher damage and DPS to compensate for this.
I'm happy the game has that back and forth that I was looking for, but I feel a better job could have been done with the passives upgrades.
Nerdy: During that playtesting phase, were there any surprising reactions or feedback from players that influenced the game's direction or updates?
Loki: Well, during the playtesting I handed the game to only a couple of people, very minimal testing outside of my own, but the most funny and enjoyable aspect of it, was to see how much they were enjoying the music. Feedback wise, I'm sure there were minute aspects that needed a look over, a quick polishing and maybe some HP reduction to the final boss. Some time after publishing, our very own Achie mentioned a small QoL [Quality of Life] feature to be able to see the player stats in-game, which was easy enough to do and now its part of the latest update the game received.
Nerdy: Its funny you were surprised by people enjoying the music because in one of my favorite Pico-View articles of yours, you wrote about how to make sure the SFX blend with the Music in games and your musical talent really shows in BTH and your more recent game (which I want to get to). But since you mentioned music, I wanted to ask at what point in the development process does music and SFX take front stage for you?
Loki: Well, thinking on it, its about the 40%-45% mark of the game. Music is integral to me, and playing the game while listening to the song and SFXs that goes with it is of high priority, since its one of two main forms of communicating with the player in a videogame —which is an interactive audiovisual medium. Thinking about it in those terms, the interactivity along side the auditory is why SFX are so important as are popups and flashes. Regarding the music, I think gameplay sets the vibe of a game, but song sets the mood, so having the music early starts to help me see the final game even while developing.
I do think I tend to do my music relatively earlier that most people, and its easily understandable why. Making games is multifaceted discipline, and as mostly solo devs, we have to wear all the hats of development, so we will focus on the ones that we can do our best on.
Nerdy: That's certainly true for me as a visual artist preferring to start projects with fully realized visual mockups and I do tend to push music towards the end when I'm near burnout. But music really changes the gameplay so I totally agree on the importance of finding the musical mood earlier on.
In your Dev Log for BTH, you admit to nearly burning out, writing "...looking at this game still stresses me some. The results are there and I'm so happy the game is out, but it was at a cost I'll consider more seriously later on." And since then you have developed and released another fantastic game, Steel Surge. Can you elaborate on what considerations you learned to better guard against burnout, and were they successful during development of Steel Surge?
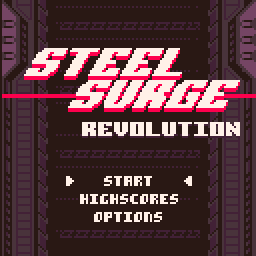
Loki: Oh yes, BTH was quite tough since it touched the Token Limit at the 92% done mark. Not having struggled with the token limit before really, it made it quite difficulty to wrap it up, but I think the aspect that made it worse was that I gave myself a deadline, a personal challenge with no real impact beyond mine. I know why I set this deadline, and while it was effective, it took its toll on me. That being said, now looking back on it, I have way more rose-tinted glasses, but I felt very real in the moment.
Steel Surge (SSurge) is different, since, in my eyes, it is an unfinished game. I know that the playable version feels complete and it was made with that intent, but SSurge in its current form is more of the first big step of 5 total. So my energy and vibe towards it is much different than BTH.
That being said, the development cycle of SSurge has been way longer and keeping focus and staving off burnout has been very much on the plate. To me, one of the aspects that have made SSurge easier to keep developing has been setting up a goal of what the game is and will be, both a promise and a direction of what the game looks like at the end of the tunnel. This, funnily enough, comes in the form of an early version of how I want the game to end, both visually and musically, so this journey is more about getting the game there rather than my projects before which has been to keep walking till the game feels complete.
Another aspect has been the very inspiring Lazy Devs Community where SSurge was developed around, and may others like Kalikan by LouieChapm were and are being made. Seeing their work really pushes you to keep working on your own game, as you want to do cool stuff just like theirs.
Nerdy: That community motivation is what I like to hear, and it's been so fun talking with both you and Louie and watching your development updates as you continue to work on your incredible shmups almost side by side.
With all that said, you've had 2 amazing releases in 2023, both easily making everyone's list of must-play PICO-8 games, what does 2024 hold for LokiStriker the game dev?
Loki: Well, definitely more games! SSurge most likely will be done in this upcoming year, but I might release one or two smaller games before SSurge is done. After that, well, with Picotron on the horizon, I'm super excited to see how that's gonna change the landscape of development of this community, and will be participating as soon as 1.0 drops (hopefully soon).
I'm strongly considering doing a YouTube channel as well, where I talk about my games, mostly from a design/development angle as well as some PICO-8 juice here and there. I'm very excited for this new year, since I feel more and more confident about my design and skill as a developer seeing how much people are enjoying my games. I hope to keep delivering better and better experiences as time goes on. And I'd love to share this as well, which most likely will be the next game I will be releasing. I guess some game ideas are hard to let go ?
Nerdy: Wow, I wasn't expecting to have ANOTHER game to look forward to! Awesome! Thanks a lot for taking this time and sharing your game dev methods with us. I'm sure we'll see a lot more of you next year. Until then, have a wonderful holiday season and a happy new year!
Loki: You as well and thank you for having me back once again! Really looking forward to see future of the Pico-View Zine as well.
Apskeppet
Year in Review
Year in Review
by Johan Peitz
In the beginning of 2023, I decided to quit a well paid job and go full time indie. I wanted better work/life balance, work on my own ideas, and build a community and customer base around my creations. Also, I had this crazy idea that it ought to be possible to make a living on PICO-8 games alone. I had some spare funds tucked away and the support of my loved ones - how did it go?
Before we get into details I want to stress that while this particular endeavor was new, I didn’t start from scratch. I’ve spent years building a presence online in general and in the PICO-8 community in particular, pushing games for free and trying to be generally helpful and sharing my work. Also, before resigning I had done some experimenting with pricing on picoCAD and picoSYNTH - and it felt totally doable to start building on that. I started Apskeppet and the journey began.
Being inspired by Sokpop and punkCake I started up a patreon. But I didn’t want to commit to making a game per month - the whole idea was to reduce stress - so I started charging per creation instead. It went surprisingly fast to get the first bunch of supporters, and since the setup guaranteed some little revenue for each title, I could bring in music and other things that I couldn’t do myself with limited risk.
During the months that passed, I managed to make and launch 5 PICO-8 games. It all started with Hellgineers, a bridge builder where you help demons cross chasms in hell. Being the debut game I wanted it to be as perfect as I possibly could make it and took a lot of time (and tokens!) tweaking and honing the gameplay and visuals. The release went well - I think? I had nothing to compare with but for a niche genre made on a niche platform with niche aesthetics it went as well as it could.
Another silly idea I had was that I wanted to make very different games. This of course meant that there was less reuse between projects, but it kept the challenges fresh and exciting. Always new technical puzzles to solve. On the flip side it made me push boundaries a little too much and I don’t know how much time I’ve spend at 8192 tokens trying to shave of a few more, or refactoring a whole system just to realize my plans.
With Shadow King I hit a brick wall both technically and creatively at one point and ended up pausing the game for a couple of months before I could return to it with fresh eyes and complete it. During that break I participated in the lowres game jam and it really helped to flush out a lot of the frustration that had built up. It also meant that I made Outbreak which was another game I could release.
Outbreak having been free during the jam of course didn’t sell much, but it still functioned as a creation on patreon, and kept building up my portfolio. Shadow King on the other hand went much better and quickly caught up with Hellgineers in terms of sales. I thought the market was ultra saturated with pixel art metroidvanias, but I guess there is always room for one more…
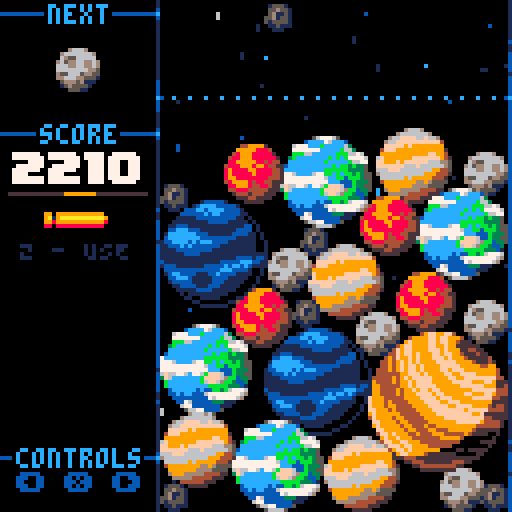
After that the Suika (Watermelon) game craze started and inspired by the PICO-8 demake, I decided to give it a whirl and make my own version of the concept. Development was fast and the game was out in a couple of weeks. Cosmic Collapse quickly caught on and was even picked up by a few mid to large sized streamers without me having to beg. Again sales quickly caught up with previous titles and surpassed them. If I could only keep this trajectory, things were looking good!
I wanted to make one last game before the year ended and decided to make a sequel to an old jam game (Golf Sunday). I had always envisioned a bigger game with more going on, and so Golf Monday was born. Bigger course, more holes, more interactions, better graphics, lots of NPCs playing alongside creating humorous interactions while trying to get a good score. Would this keep building on my previous successes? Short answer - nope. The game fell totally flat. People playing it really enjoyed it but I could not manage to build up any type of wider interest or hype around it.
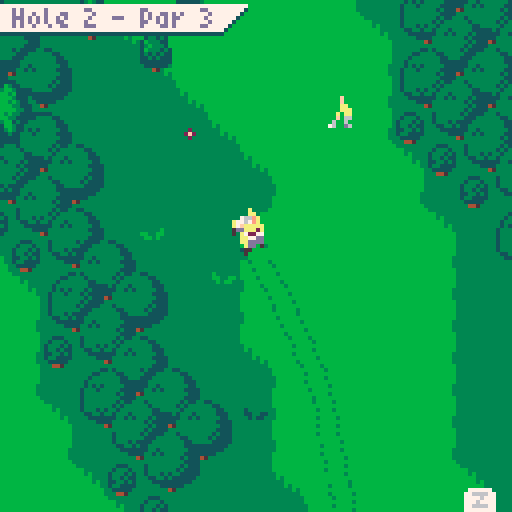
So with almost a year as solo dev and 5 commercial PICO-8 games released, what are my key takeaways?
- I did what I set out to do! I’ve worked less hours, but more focused. I’ve spent time on games I want to make myself, without caring too much about the market. And I started building a community on discord. It is small, but it is growing.
- Patreon is a good base for guaranteed revenue, but it is only a fraction compared to direct sales.
- I was planning to use Twitter/X as my main marketing tool. It worked very well in the beginning but as the platform started its decline it made a major dent in my plans. Still trying to recover and find new ways.
- Do I really have to break new ground every single time? Who actually (beside me) cares about the final polish touches I spend so much time on, and more importantly does it affect sales?
- Among the most enthusiastic players of my games were handheld owners. I’ve seen so many cool pictures of my games running on hardware.
- Games can make revenue in many ways! A school bought hundreds of licenses for Hellgineers to use in class.
- Steam and itch are different platforms. If I do zero marketing, the games still sell on Steam whereas they fall into the shadows on itch. Of the games I’ve put on steam, they seem to sell 2-5x better.
- I suck at marketing! I don’t enjoy it, and I don’t set aside time for it. My estimate is that I could “easily” double the revenue with a little more effort.
- picoCAD keeps selling. What a time to be alive.
So what’s the end result - am I running out of funds or is it working? The answer lies somewhere in between. Some months I’ve had crazy record sales while others have been shockingly slow, but overall it looks like an upwards trend. Some months I break even, some months I don’t. The revenue I’m getting is definitely making my savings last longer, so I’ll keep going a little more.
Going into the new year, I’ve been thinking a lot about what I want to keep doing, and what I might want to change. I want to keep making small games, but more often than not I’ve felt the constraints of the PICO-8 platform holding me back. I’ve been experimenting a bit with love2D and I can definitely see myself using that for some projects, and sticking with PICO-8 for others. It is also clear that I need to step up my marketing game. I can’t make more games per hour, so I need to sell more games instead. Lots of room for improvement here.
As a last note I just want to reach out and say thanks to everyone who has been cheering and supporting my new journey. And even if things go south down the line I’ve at least had a ton of fun trying it out, been less stressed, and spent more time with my family.
Curated PICO-8 Games Library
by NerdyTeachers
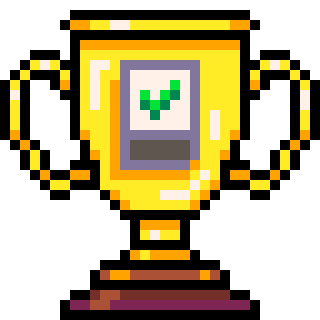
Last month, we were proud to announce the Top 200 BBS rated PICO-8 games since 2015. It is already being used well to help gamers quickly discover some of the best PICO-8 experiences on the fantasy console. If you were not aware of that announcement, check it out here:
Top 200 Games
But that was just a taste of what we were actually working on!
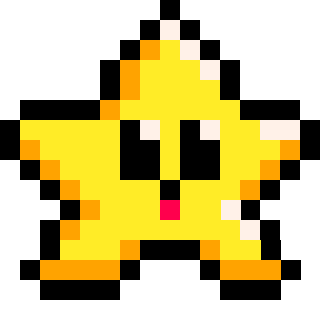
The Bigger Announcement
To celebrate 12 issues in 12 months, and as a New Year's present to all, we are very happy to be able to release this project packed full of features! We have sorted through the entire BBS (~15,000 carts), cut it down to only carts that received 10 stars and above (~2,000 carts), and have been continuing a curation process to find and properly label the ones that are polished, complete, and fun.
What Labels?
When a cart is uploaded to the BBS, you give it certain information that labels it for easy searchability such as a title, Creative Commons license, and tags. But we'd also like to know a few more things about the game, and we've been adding these manually, one by one.
When you browse a cart in the Curated List, you can hover your mouse over the icons to learn what these labels mean and quickly understand more about the game.
1. Player Count
2. Cart Count
3. Remake/Demake
4. Full keyboard and mouse
5. Creative Commons
6. Genre(s)
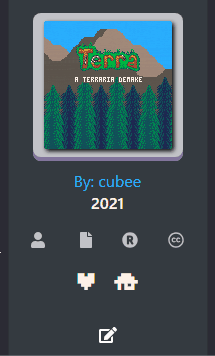
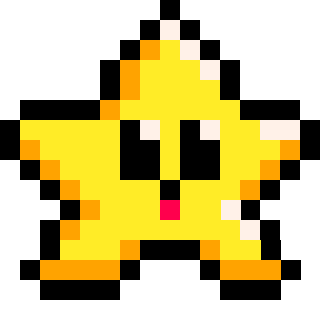
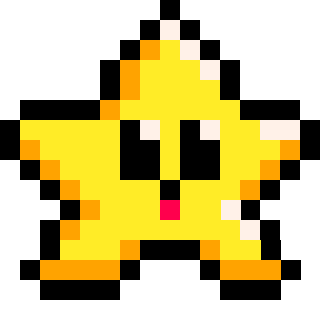
The Features
To really get full use out of this curated list of games, it is important to be able to search, filter, and sort through the list in many ways to discover exactly the games you will more likely enjoy.
Search and Filter by: (all optional)
Keyword | One or more words to find in title, tags, or developer name. |
Genre | A descriptive categorization of game. (platformer, shooter, etc.) |
Player Count | Single player, multiplayer, and up to how many. |
Year | The year the game was released. |
Controls | Default or Full keyboard and mouse support. |
Copyright | All games or only Creative Commons Licensed games. |
Second Genre | Another genre to narrow the type of game. (action+strategy) |
Sort by | Display the results in order of popularity, title, author, or date. |
What are the Genres?
Game genres are difficult to define, and categorizing games is far from an exact science. We researched quite a few different game library sites that all had their own definitions on the common genres we know. Some genres are umbrella terms such as "Shooter" that has even more commonly used sub-genres within it: "Shoot 'em up", "First Person Shooter", etc. Some genres are a blend of two general genres to create a specific one such as "Action-adventure", "Puzzle-platformer", etc.
So we took all this information and distilled it down into what we are happy to use here. Genres here are not categorized boxes that a game must be sorted into. Instead, our genres are simply descriptions of a type of gameplay, style, and/or mechanics that can apply to any game. We also had fun limiting ourselves to the set of PICO-8 glyphs to represent the different genres.
A couple of these are probably unexpected like, "highscore" (points based tracker) and "speedrunner" (time based tracker). But because these genres are meant to be descriptions that you can search by, including these type of labels just made sense.
If you are wondering how exactly we are defining and describing these genres, well we made a page just for answering that question.
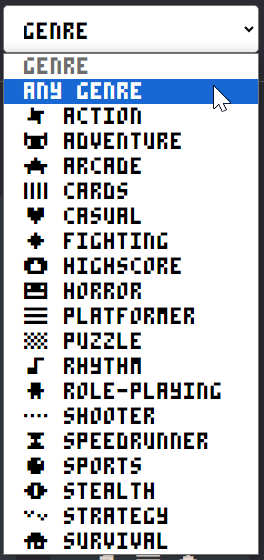
Lucky Draw!
You may have noticed a small checkbox on the right side of the additional Search Filters in the image above. This was a requested feature for any of you who just want to be happily surprised with a shortlist of games that you can jump into and play without the distraction of a potentially long list.
By checking the Lucky Draw box, you will only be presented with 3 random game results.
The best part? This is still tied into your other search criteria! So leave all the other options blank to get just 3 random games from our entire list. Or you can make your search as specific as you'd like, for example:
3 Random Platformer Games!
3 Random Two-player Action Games!!
3 Random Highscore Shooter Games from 2023!!!
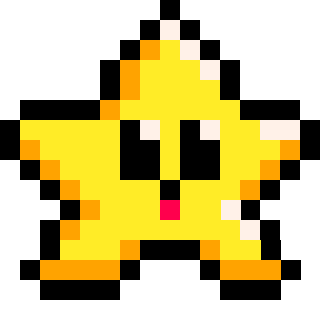
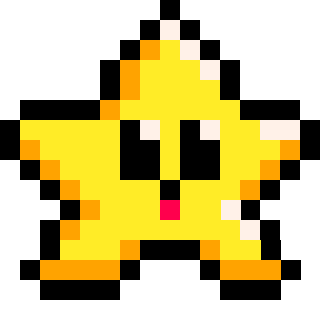
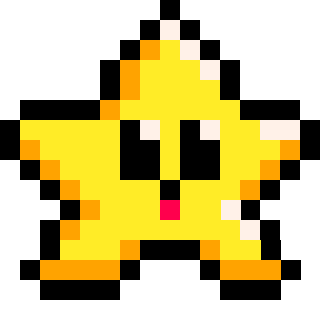
Community Curated
This started out with a much smaller scope, with the idea of having a few trusted curators that will edit and add to this list slowly according to our own judgement and preferences. However, it has already outgrown that!
We decided to immediately allow everyone to help us curate the games in the list and let it be a real community driven effort. So underneath each game listing you will see a "Help Edit" button.
Click on this to open a popup where you can edit or add to the labels attached to a game.
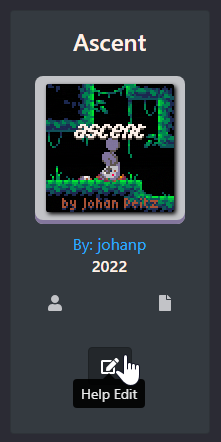
After clicking the pencil icon at the bottom, you then simply add to this pre-filled form and send it in to be verified and applied to the game!
You won't see the changes applied until someone from our team has had a chance to verify the new data, so go nuts and send as many game edits as you can! It will really help us speed things up.
Note: sending suggestions for the same game multiple times will be lost as spam.
Flag Games
Another feature in this Edit Suggestion form is the ability to Flag a game. If you click on the red Flag dropdown button on the bottom left, you will see 3 options: (1) Poor Quality, (2) Demoscene, and (3) Tool.
Flag | When to flag a game |
---|---|
Poor Quality |
You feel this game is unfinished, unpolished, or just not up to the standards of quality and fun as the rest of the list. |
Demoscene |
This is a neat cart with impressive visuals, animations, music, or code but not a full game. |
Tool |
This is a cart that is meant to help others make games in some way, but not a game itself. |
Flag suggestions will not be applied immediately but after verification, they will be removed from the list of Curated Games. However, as the list of carts flagged as "Demoscene" and "Tools" grows, we may be able to open specific pages to browse for those specifically. So let us know if that is something you'd like to see in the future.
Final Feature: Nominate a Game
If you ever want to bring a BBS game to our attention that you feel should be included, then there is always a small link for that on the left side of the page. It will also appear as a larger button if your search returns no results.
You can see a second button that will take your search criteria, and bring you directly to the same search on the BBS. Although, the only search options availble to translate over are the main genre as a single tag, the keywords, and whether you specify for CC licensed only games.
The nomination form is very simple with just the title of the game, a link to the BBS page, and your reasons for nominating it.
Final Disclaimer: Because of the interactions and forms on this page, we did need to take some precautions such as for spamming, so even though it is still completely free to access, we do require a Google login, same as our Sprite Library.
Have fun browsing PICO-8 games like never before!
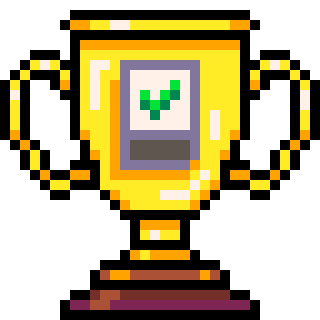
Curated PICO-8 Games
Outstanding Outliers
Speedrunning in a Winter Wonderland
by KumaKhan22
The month of December is celebrated by most every culture on the planet. Tradition is the watchword for the last day of the calendar year and one of the most ubiquitous rituals is the watching of classic holiday movies. Everyone has their own unique list, but two films are unanimously (some say infamously) included on every watch list.
“Home Alone” was an immediate cultural sensation when the film dropped in 1990, combining a very strong cast of actors with brilliant direction, production, & writing; throw in John Williams doing the music and an evergreen holiday classic was born. Even with all these wonderful pieces coming together, they still needed a powerful bonding agent to make it all work; that agent was Macaulay Culkin. One of the most successful and recognizable child actors of all time, the kid in all of us immediately identified with the loveable little one as he struggled to be seen & heard by his family only to find himself in the middle of an increasing amount of trouble and danger as the holiday approached.
The franchise made the most of its box office success by releasing several games across multiple consoles within a year of the film's release. They all share the same goal of collecting valuables in the McCallister house or neighborhood to thwart the burglars who are set to steal everything that isn’t nailed down. While most of the games have similar level design & win condition involving boss battles, it’s the first game on NES which is an outlier among the rest.

When you power on the game and press the start button at the title a 20-minute timer begins off-screen, then all the player must do to win the game is avoid being grabbed by one of the two burglars until the end of the countdown. Clever speedrunners have already found a way to break the game by setting up a bug in the software, guaranteeing a victory without playing the game for more than a minute; this has led mobs of streamers to learn this glitch and as a result the World Record for this game is held by, as it stands after this holiday, over 250 individuals; 15 of those being new runs just this month alone. There have been live marathons that encouraged groups of people to achieve this record simultaneously and small gaming communities that run this game every year; the list keeps growing, maybe next year you the reader will join in on the holiday fun!
The next most talked about movie that crosses the bounds of being a holiday classic is none other than “Die Hard”. This one is argued about to the point of banality whether it is a Christmas movie or not, and really it comes down to a simple matter of opinion rather than there being a right or wrong answer; in this writer's opinion it IS a Christmas movie, but it’s not meant for kids. The most compelling point I can make is the amount of holiday music featured in the film and on the soundtrack; mixing traditional tunes like “Ode to Joy” with contemporary jams like “Christmas in Hollis” and other holiday flavor-ites sets the holiday mood of the movie.
Some circles even regard this movie’s main hero & villain as being parallels of those from an older, more certain holiday hit film, “It’s A Wonderful Life”.
While the “Die Hard” series would continue to attract movie-goers, the license was directly loaned to several games in the early 90s. Among them, the NES title has seen a lot of attention in the speedrunning community, however not nearly as much as “Home Alone”; though every December a new batch of runs roll in for verification.
Diverting from this game I would like to suggest what I consider the superior title that came out later in the series, “Die Hard Arcade”. Aside from being a quarter-eating cabinet it was ported to Sega Saturn & PlayStation 2. The home console port still has the quarter-eater level of difficulty, so to balance this the player is allowed to challenge a separate game called "Deep Scan”, where you can sink submarines to earn credits towards the main game. Due to licensing issues, it was released as an original property in Japan where it was called “Dynamite Deka” and did receive a proper sequel, though the series continued to have an original story in Japan.
No matter what movies are on your list we can all agree that there is no more comforting thought this time of year than curling up comfy on a couch with a friend or loved one, enjoying some nice snackies & drinks, then traveling back in time with your favorite films.
Happy holidays everyone and a prosperous new year to us all.
Pixel Art Gallery and interview
Artist Spotlight: SaKo
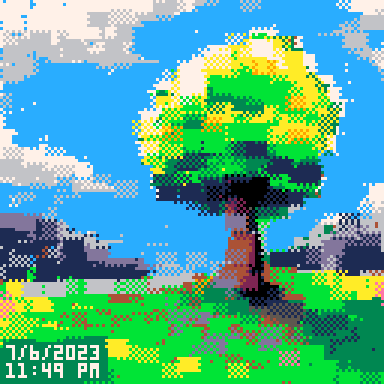
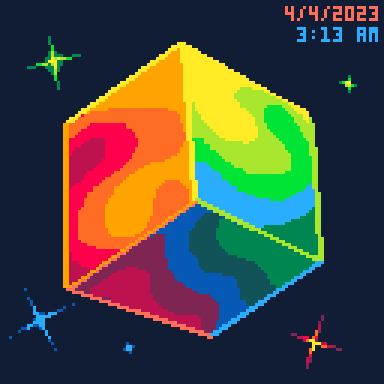
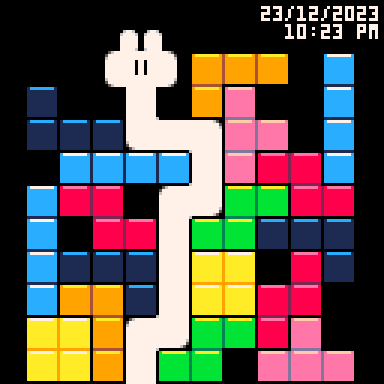
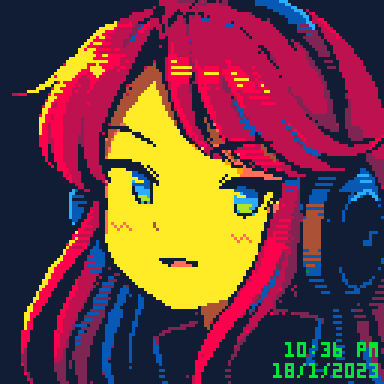
Editor's Note: As we have been reflecting on the entire year in this issue, it was fitting to find a pixel artist who has dedicated every day of 2023 to making art. If you are unfamiliar with SaKo, they are a game developer and a student of Electronics Engineering from Perth, Australia. They made their own drawing tool within PICO-8 that has been available to supporters on their Ko-Fi (public release soon), and all of their art you see here was made on that with the PICO-8 Palette.
We reached out to SaKo very near the end of this year as we were getting more and more excited to see them approach the achievement of 365 drawings in 365 days. After following their journey all year, I was full of questions which I dropped at their feet all at once, and they were gracious enough to take the time to respond to create this interview:
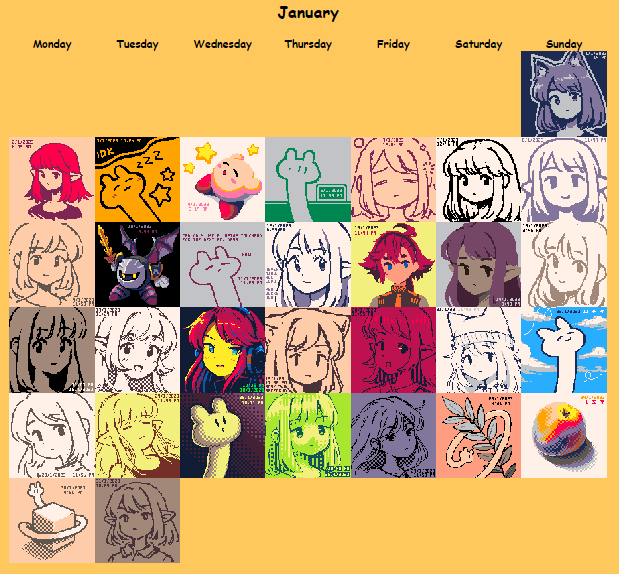
Nerdy: What made you decide to start the Daily Doodles for the entire year?
SaKo: I didn't draw very often previously, I would sometimes spend weeks or months without drawing anything or posting any art. I felt like I wasn’t drawing enough and wanted to push myself to draw regularly, so late last year I decided to try drawing with a pen every day in a small 2022 diary/planner book. I can be somewhat of a perfectionist so drawing with a pen forced me to keep moving on and not get caught up in constantly erasing and redrawing things.
I also started learning PICO-8 last year, with Lazy Devs Academy’s excellent shmup tutorial and their other videos. Near the end of the year, I started developing my PICO-8 drawing app. I was inspired by others who made their own creative tools from scratch and posted art or music made with them. I've always wanted to try doing the same thing and make my own program to create art with. Amidst the growing turmoil and anxiety around art at the time, and fuelled by my passion for human-made art and my growing confidence with PICO-8, it felt like the perfect time to try making my own silly little drawing app.
As 2022 was ending, I wondered if, and how, I will continue the doodles in 2023. As a primarily digital artist, I liked that doing them in pen on paper pushed me out of my comfort zone. However, I quickly realised that this was the perfect opportunity to put my drawing app to use. If I drew in it every day, it would motivate me to keep working on it and add new features. I thought it would be a fun challenge and as someone who used to post art very infrequently, I liked the idea of having something to post every single day.
Nerdy: We get to see so many fun variations of your original characters. Can you tell us about their background story and has their story evolved over this year of drawing them?
SaKo: The long character with the big, bunny-like ears, I call them Creature (or, the creature). They have an infinite/unknown length body that defies space and physics. They started as a quick scribble I drew some time in 2021 and didn’t think much of. Later, I was looking for something to use as a new profile picture and thought the creature I drew was kinda funny and cute, so I decided to use it. Over time, the character grew on me and now I guess they somewhat represent me. Sort of like my mascot or something, I don’t know.
I didn't really have my own original character that I drew often before. I used to pretty much only draw fanart or characters I design on the spot and then never draw again, so it was nice to finally have my own character I can doodle easily. Their original design is a bit… different to how I draw them now. I made them more round and smooth and adjusted their proportions over time, though I think the cursed (in my opinion) energy of the original is funny.
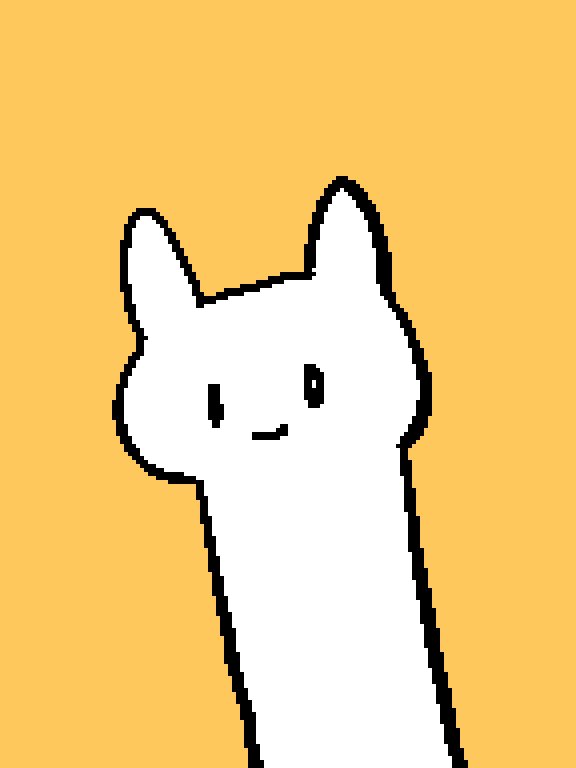
I haven’t given them any “lore” or anything like that. They’re just an infinitely long cartoon creature, that’s it. What I like about their simple design and lack of backstory is that I can draw them in any way, doing anything. If I couldn’t think of what else to draw (happened very often), I could always draw the creature. If it was almost midnight and I was running out of time (also happened very often), I could always scribble the creature. That’s what led to me drawing them so many times lol.
As for the human (or human-ish) characters I’ve drawn, they’re all designed on the spot, though some of the early ones were fanart. If some of them look similar to another, it’s just because of my style (and I keep drawing the same few hairstyles that are easy for me).
Nerdy: What was the biggest challenge or difficulty to staying dedicated? How did you push through to keep producing daily?
SaKo: I didn’t post my 2022 pen doodles publicly at the time, but I posted them weekly for my Ko-fi supporters. Maybe because they were semi-private and posted weekly instead of daily, I slacked off a lot and did not actually draw every single day. I had to keep catching up on the days I missed before posting them each week.
So, one of the reasons I decided to post my 2023 doodles publicly was to hold myself accountable and push myself to actually draw every single day. Despite my slacking off in the previous year, I was confident that I could keep up, as I’ve gotten used to following a daily checklist for a few years now and this would just be one of the things to check off each day. Since I’m drawing in an app I made myself, I took advantage of that and made the timestamp tool, so that I can be transparent and add context to every doodle. Timestamping every doodle pushed me further to make sure I finished each one on their respective days. You can see that for many, maybe even most of my doodles, I rushed to finish them before midnight lol.
I also started a full-time electronics engineering course this year, and for some reason I did not anticipate the workload and how tired I would be at the end of each day. There were a few times I wanted to take a short nap or just fell asleep before drawing for the day and ended up waking up after midnight. That’s why some doodles are timestamped as the next day. I also intended to keep working on the app throughout the year and regularly add features to it, but I ended up being so busy with the course and other things that I didn’t have the time, so I wasn’t able to make the app as featured as I wanted to by the end of the year.
Maybe the biggest challenge was the rush to finish each doodle before midnight. Figuring out what I can draw that I haven’t already drawn yet, and then racing against the clock to finish it. Sometimes I would manage to come up with a fun idea and draw something I’m happy with within the time limit, but often I had to just scribble *something* and get it out the door. Maybe I shouldn’t have kept leaving it to the last minute, but it’s part of my nature I guess. Having the creature I could always scribble when I was out of time and ideas was very helpful haha.
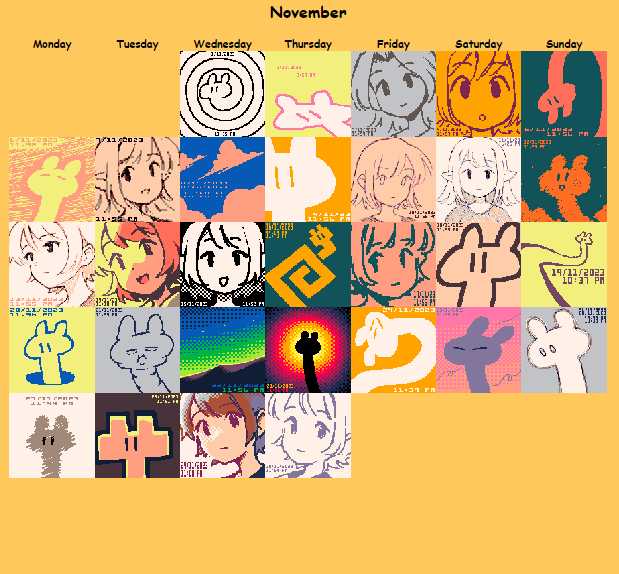
Nerdy: Now that it is coming to an end, how does it feel to reach such a long term goal and how has it benefitted you as an artist?
SaKo: As someone who really struggles with finishing big projects, it feels great to reach the finish line of this somewhat ambitious year-long project. Both because of the accomplishment and also because I’ll have one less thing to do each day lol. I’m very happy that I was able to stick to it for the entire year. Each doodle took only a few minutes each day on average, but adding them all up, plus regularly posting them to multiple social media websites, plus making the pages for my website and updating them every month, it took a lot of time and effort in total. So far, this might be the longest and most ambitious project that I’ve actually been able to finish, so that’s pretty cool!
I’m not sure how much my skills have improved throughout the year, as most of my doodles were very simple and very few of them had me pushing my skill boundary. I very much stayed in my comfort zone for most of the doodles. I think what got the most benefit was my mindset. No matter how “bad” a piece might be, if there was no time left, I had to post it. It felt embarrassing at first to post art I wasn’t happy with, but I got used to it over time. Eventually it became a neutral “it is what it is” feeling whenever I had to post some rushed scribble. It might sound weird to say that’s a good thing, but this is the mindset I need if I want to finish projects and get them out the door. I really hope I maintain this mindset for the future!
Nerdy: What do you have planned for 2024?
SaKo: This was a really busy, somewhat stressful year and I spread myself quite thin, so my plan for next year is to focus more on fewer things. I want to be able to focus more on my assignments and after I finish the course, have more time to work on bigger projects. I probably won’t be doing anything like this challenge next year. I want next year to be more quality than quantity for my work.
I am also really excited for Picotron! It might be my most anticipated upcoming release out of everything. I’m definitely planning on making stuff and experimenting in it when it releases. I say this every year, but I hope I can finish and release a small game next year in PICO-8, Picotron, or on the Playdate. Gotta make a game in Lua on something that starts with a P. (I’ll also probably be experimenting with Love2D. If only it also started with a P, oh well!)
The Past and Future of Pico-View
by NerdyTeachers
I honestly cannot believe an entire year has passed. I am incredibly proud of what we have achieved when I look back on all 12 issues of Pico-View. It was a giant effort only made possible by the incredible authors and artists who were willing to teach and share. Our goal became one issue a month for an entire year, and...We did it!
Honestly, I had a completely different plan when I started this year. I announced my return on Youtube with an overhaul of the website, our Discord server, and our API guide. That was the plan, finish the guide to make written tutorials easier, to make video tutorials easier. Well the guide still isn't finished, and we didn't make more videos on Youtube. What happened??
Pico-View Origins
Marina, who I credit as the true founder of the zine, was one of the very first to join our Discord, and she had already done a few interviews in 2022 with devs in the PICO-8 community as posts on the BBS —PICO-8 Interviews that she called "Pico-Views".
I was a big fan of that content and she mentioned a desire to expand it in some way, so since I was already restructuring NerdyTeachers.com, I had an idea. "What if we host your interviews on here?" I proposed, "so you can have a single page where people can view all of your interviews. Just like our sections of tutorials, you can have a section of your own."
She loved the idea and we ran with it. I set up a section for Pico-View, gave her access to add and edit the pages like a blog, and I thought I would simply make my own content over here and she'd do her thing over there. Well, when I say we ran with it....that first month Marina really ran, much much farther than I thought she would!
Over the year I've become good friends with Marina and gotten to know her well. She often gets intensely excited over grand ideas that suddenly inflates from a handheld balloon to a hot air balloon over the course of a couple sentences XD. The excitement is contagious to say the least, and in January, our small plan for an interviews section grew and grew into this complete webzine!
The January issue was entirely her making, and we've been following many of her precedents since; from Cover Art, interviews, reviews, to articles on game dev. She was gathering all of her friends in the community to contribute in some way.
It was hard for Achie and I to resist getting swept up into this exciting endeavor, but we both stayed reasonable and offered small but consistent parts to play. Achie would do one big game review, and I would make one simple prototype.
When Marina took lead, she compiled and posted the zine to the BBS, and I would reformat it over here with some HTML/CSS benefits like internal links for a table of contents, code snippets, centered images, columns, etc. After February, Marina was definitely getting burned out, and Achie and I began stepping in to take over more duties and share the load of coordination, and that sort of snowballed from there. The zine itself also snowballed in size and readership which was incredible to watch.
We knew we were doing something special and had to keep it going. I already set my own goal of wanting to see Pico-View through all 12 months of 2023 and that often kept me going through the multitasking stress. It was that goal that pushed me to take on more responsibility and step in when anyone had to step away. It was tough, and it took priority over my original goals, but I'm really happy with what we did instead.
What's Next?
In 2024, I will definitely be returning to my original goals of completing the Guide pages, writing many more tutorials, and making many more tutorial videos on our YouTube channel. We also of course want to maintain and update the new Curated PICO-8 Games List. My ultimate goal is to bring PICO-8 into more classrooms around the globe by making it as easy as possible for students and teachers to learn and use.
However, I don't want to completely drop Pico-View to do those things, so my plan is to continue whatever amount I can to bring more news and articles to you, keeping this space open to devs who are happy to share, and spotlighting amazing games, developers, artists, and musicians in the community.
You can help us do all of that next year by becoming a supporter. We currently have a goal of $500 and the plan is to release the next issue each time we reach that goal. By becoming a supporter of the higher tiers, you'll gain early access to the content we are working on before we reach the goal and release it publicly.
If we reach the goal this coming month, then Pico-View is guaranteed to continue on schedule with a January 2024 issue! If we don't reach the goal, and instead for example we get half in monthly memberships, then that means we will reach the Pico-View goal every 2 months, and so Pico-View will become a bi-monthly zine, releasing every other month in 2024.
Click the blue "Support Us" button in the lower left to become a supporter. Both one-time donations and monthly memberships will count towards the Pico-View 2024 Goal.
Thank you all very much, Happy New Year and we'll see you in 2024!
Closing Remarks
Thank you for reading the December issue of the Pico-View web-zine! We hope you enjoyed all of the articles this month. Here are the folks who helped piece the zine together one pixel at a time...
-Zep - Cover Art
-Tubeman - Article Writing
-LouieChapm - Interviewee
-Lokistriker - Interviewee
-Johan Peitz - Article Writing
-KumaKhan22 - Article Writing
-SaKo - Interviewee and Pixel Artist
-Achie - Game Reviewer, Article Writer, and Zine Coordinator
-NerdyTeachers - Editor, Article Writer, Interviewer, and Illustrator
Thanks to all the authors, contributors, and readers for supporting our PICO-8 zine! If anyone would like to write an article, share pixel art, or help with anything contact us at @NerdyTeachers on twitter or Discord.
-Nerdy Teachers
12811
26 Dec 2023