pico-view:
August 2023
Hello Pico-View Reader, this is Marina, and this August issue makes the eighth in the series. I started Pico-View because I wanted a place to come for monthly pico-8 content, and I believe my friends here have accomplished that.
I'm happy to say that our little zine has grown a great deal due to the contributions of Nerdy Teachers. This will be the last issue that I will take a lead-role in the creation of, although in truth Nerdy has been the organizer for sometime now.
I thank him and you all for your continued efforts and contributions on Pico-View. I truly appreciated my time working on the zine, so I thank you all for that as well. Without further ado, have fun reading this month's Pico-View!
Authors:
Fletch, c.diffin, Jammigans, Josiah Winslow, D3V?, PBeS Studios, Alethium, Lucas Castro, SIC_Benson, Liquidream, Pico-8 Gamer, Sign, PJBGamer, Marina, Achie, and Nerdy Teachers
Contributors:
Wolfe3D, Pancelor, Street-Monk-1255
Contents:
-Cover Art by PJBGamer
-What is a Lerp? - Fletch
-Making Music with Loops - c.diffin
----------------------------------------------
-PICO-butter and Jam 2 - NerdyTeachers
--------------------
-AGBIC Jam
-Apathello by Josiah Winslow
-Going Astray by D3V?
-Homunculus by Nerdy Teachers
-Kumatori Panic! by Achie
-Kumatori Panic! by PBeS Studios
-Order Up! by Alethium
-Peas! by Lucas Castro
-Runaways by SIC_Benson
--------------------
-Low Rez Jam 2023
-Low Rez Jam PICO-8 Games
--------------------
-1-bit Jam
----------------------------------------------
-Featured Interview - Jammigans, Fletch, Mothense ft. Marina
-Featured Game Review: Occult Gunner - Achie
--------------------
-Upcoming Jam: PICO-1K - Liquidream
-Upcoming Jam: Cre8 - Pico-8 Gamer & Sign
--------------------
-The Death of a Frog - Marina
-Random Reviews - New Release Recommendations
-Pixel Art Gallery
-Prototype Party
-Closing Remarks

What is a Lerp?
by Fletch
The word "lerp" is just an abbreviation of the phrase "linear interpolation". For our purposes, linear interpolations (from here on out, lerps) give us the ability to represent change over time. These mathematical functions allow us to cheaply animate or change any values we want in our code!
For PICO-8, this is awesome, because animation engines can often consist of complex state machines or heavy easing engines. If you want something slimmed down and quick to use, try out a lerp!
How do they work?
Lerps can look differently depending on who you ask, but I am personally a big fan of lerps that take in three parameters; the first two being the most important: from
and to
. The question we want our lerp function to answer is: "How much should I change from where I am now to where I want to go?"
Let's take a look at the simplest of lerps - we'll just add a static value to our position every frame. Here's what the lerp might look like:
function lerp(from, to, distance)
if (from < to) then
return from + distance
end
return to
end
In plain English, this function will take in three parameters: from
, to
, and distance
. We will determine if we are already in our final state. If so, we'll just return to
. However, if we haven't yet reached our final state, than we'll add distance
to our current value from
to get a little closer to to
.
Using a square's X position on the screen, this lerp might look like this:
Notice how this lerp has harsh motion. It starts and stops abruptly, and the motion in the square doesn't carry much character with it. We can spice it up a bit by using a weighted lerp! Here's the code for that:
-- weight must be between 0 and 1
function lerp(from, to, weight)
local dist = to - from
-- if we are "close enough" to our target, just move to the target immediately
if (abs(dist) < 0.2) then
return to
end
return from + (dist * weight)
end
You'll notice that in this lerp function, the parameters have changed. from
and to
are still with us, but now we've swapped distance
for weight
. In plain English, this function will determine the distance we have remaining to reach our to
value, then change from
by a fraction of that distance.
The fractional distance we move each frame is determine by weight
! If we provide a value of 0.10
, the lerp will return a value that is 10% closer to our final value. If we provide a weight of 0.52
, the lerp will return a value that is 52% closer to our final value. Here's what that looks like:
You can see that this animation starts out fast, but as we get closer, we slow down. This type of motion is great for making characters, menus, and more have a lot of charm and appeal to them! Try to find all the ways we use weighted lerps in our latest game, Canyon Crisis:
Applications of Lerps
While lerps are great for positional movement, they can be used for SO MUCH more. Since they just represent a change in a value over time, we can use them for all sorts of things!
What if you want to cycle through a set of colors? Sure! Create a table of colors, and then iterate through that table with a lerp and an index.
-- init
idx = 1
target = 9
colors = {2,8,4,9,10,11,12,13,2}
-- update
-- not a weighted lerp - distance is 1 so that we move to the next table index
idx = lerp(idx, target, 1)
-- draw
rect(28, 28, 36, 36, colors[x])
Say you want to create a screen transition where you fade from one color into another. You could create a table of fillp()
patterns, and then iterate through that table using a lerp and an index to create the transition!
-- init
x = 1
xtarget = 9
fills = {
0b0000000000000000,
0b1111000000000000,
0b1111111100000000,
0b1111111111110000,
0b1111111111111111,
0b0000111111111111,
0b0000000011111111,
0b0000000000001111,
0b0000000000000000,
}
-- update
-- not a weighted lerp - distance is 1 so that we move to the next table index
x = lerp(x, xtarget, 1)
-- draw
fillp(fills[x])
rectfill(0, 0, 63, 63, 0xc1)
What if you have a multi-value vector, such as a Vector2
from an engine like Godot or Unity? If you're representing Vectors in PICO-8, no problem! A lerp on a Vector is the same as a lerp on it's components. So from
and to
can be tables holding x
and y
values:
-- lerp on a multi-value Vector (generalizable to Vector3, Vector4, etc)
function lerpVector2(from, to, distance)
return {
x=lerp(from.x, to.x, distance),
y=lerp(from.y, to.y, distance)
}
end
Conclusion
Hopefully you can see how powerful lerps can be! Creating motion can be simple and only occupy a few tokens if you'd like. Also, try to dream up your own mathematical functions to put inside a lerp! There are lots out there that can represent different sorts of motion, called "easing functions" One great resource for this is Easings.net.
Hope you enjoyed this quick look at lerps! Now go out there and be awesome <3
- Fletch

Making Music with Loops
by c.diffin
Hey there Pico-Pals! I’m Chris —I’m pretty new to coding and game dev, but I’ve been a hobbyist musician for a while. I just finished composing for an update to the excellent haiku-making game “Will The Man Get Frog” by Marechal Banane and figured it would be interesting to share one of the techniques I used to pack 5 tracks in to one cart!
We’re going to use loops of different lengths to create music that evolves over time and takes a long time to repeat, meaning you get lots of musical value from your patterns and SFX. Experienced musicians might already be aware of these techniques, but if you’re still getting started with adding music to your games then have I got a treat for you!
Welcome to Loop Camp, Cadet
Let’s talk about how loops work in Pico-8, because there’s two levels of looping to consider. The first is at the Pattern level, the ones you set in the Pattern editor with the buttons in the top right. !
At the moment a pattern finishes, it checks those flags in memory and decides what pattern to play next. Easy.
The other is at the SFX level. Using the number fields at the top right of the SFX editor, you can choose which step of the pattern will be the last in the loop, and which step it will go back to after that. Note, an SFX always starts playing from step 1 when the pattern starts playing, regardless of the loop settings.
This means that we can set up a pattern with multiple SFX of different loop lengths. When we play them back, we’ll get an effect where those musical phrases overlap and shift in phase over time.
Jumping Through Loops
This all makes sense, but we need to understand one more thing; when will our pattern end and check its own loop flags if all the SFX have different lengths? What if all the SFX are looping?
We can use this logic to understand when the pattern will end:
- If all the SFX are set to loop, the pattern ends as soon as any of the SFX have played 32 steps in total.
- If at least one SFX is set without a loop, the pattern ends as soon as any of those SFX end.
This can lead to some unwanted outcomes. Say we have a pattern with these SFX:
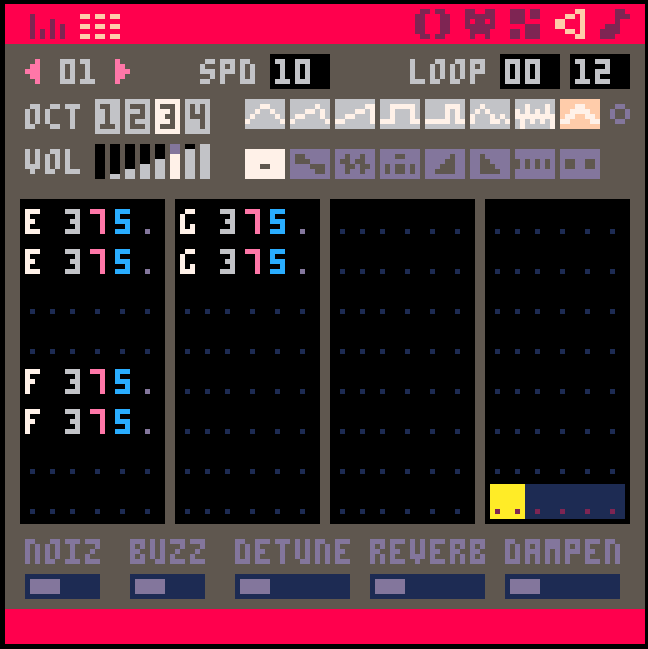
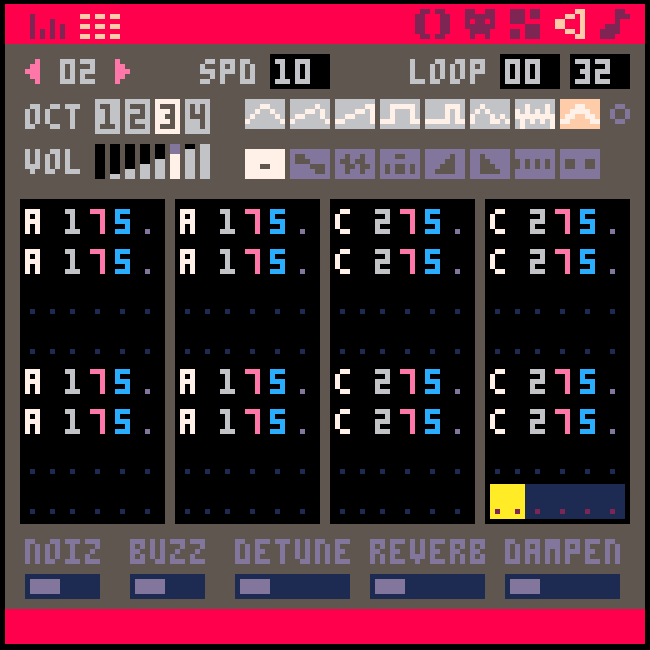
Our first SFX has 3 notes that loop every 12 steps, and our second has 2 notes that repeat over 32 steps. The pattern will end after 32 steps so this is what we hear:
SFX1: E/F/G/E/F/G/E/F/ ||
SFX2: A/A/A/A/C/C/C/C/ ||
At the end of this pattern, SFX 1 is partway through its phase. If we looped this pattern with its loop flags, that phase will reset every 32 steps and we’ll hear those same 32 steps over and over. Not what we want! Luckily there’s a hack. If we add SFX3 to the pattern and set its speed to 30 (3x the speed of SFX 1 and 2), it will keep the pattern going much longer, even if SFX 3 has no note data in it. Now when we play the pattern we hear this:
SFX1: E/F/G/E/F/G/E/F/ | G/E/F/G/E/F/G/E/ | F/G/E/F/G/E/F/G/ ||
SFX2: A/A/A/A/C/C/C/C/ | A/A/A/A/C/C/C/C/ | A/A/A/A/C/C/C/C/ ||
After 3 bars, we see that the phase of the musical phrases realign and from here we will start to hear actual musical repetition. Of course, this is a basic example and it’s possible to create phrases with much longer periodicity. The length of time before a musical repetition occurs depends on the ratio of step lengths between each of the phrases. (Hint: prime number step lengths go hard!)
Reaping the Froots of Our Loops
So why worry about this? Why not just write out all your music step by step, so you get complete control at every stage? Well, there’s two main reasons I’d consider.
The first is a very Pico-8 reason, which is that it’s very efficient. Think about your loops as functions that you get to reuse. By arranging them so that they phase against each other, you can program music that evolves and changes over a long time. Depending on your relative phasing, using only a single pattern and 4 SFX you can define music that never actually repeats itself even after 5 minutes or more!
The other is a more artistic take. One of the joys of this method is that when you’re setting up your loops and their different lengths… you might not know what the result will be! You might have an idea, especially once you have a bit of experience, but even the smallest changes can lead to massive differences in output! This lets you approach composition very differently. You don’t necessarily have to know what you want when you start- just listen to the output and make tweaks until you like what you hear!
This idea is by no means new. When J. S. Bach did it, people called it “counterpoint”. Modern composers call it “polymeter”. Indonesian gamelan players have been working with these forms for thousands of years. All this to say there are plenty of resources out there if you want some inspiration.
Here are my tips for making the most of it in the Pico-8 tracker:
- Your SFX loops can be anything! Simple arpeggios, bass note progressions, melodies and countermelodies… it also works well with drum patterns.
- When you’re getting started, try separating your melodic SFX across different octaves. This will make sure you avoid any really ugly dissonance. Listen, tweak and experiment!
- The “Control SFX” we use to set the pattern length doesn’t have to be empty. Turning up the speed value on one of your existing SFX might lose you some “rhythmic resolution” but can free up a whole other SFX slot! To that end…
- You can use SFX instruments within your loops to nest more step information within steps of your Control SFX. Using SFX instruments is its own topic, but you can get some very advanced results this way.
That’s all! If you want to hear what I did with it, check out the cart for “Will The Man Get Frog”. Here is an example of pattern 2 playing where you can see SFX 16 is the "Control SFX" (above the cursor) as it moves very slowly and sets the pattern loop length, while SFX 17, 18, and 19 are looping at their own speeds multiple times before the whole pattern completes:
Thanks for reading- find me on discord as //Chris (c.diffin) if you’ve any questions.
- c.diffin

Pico-butter and jam 2
by NerdyTeachers
Back in May of this year, we had a jam-packed month of game releases thanks to multiple popular game jams occuring at the same time —Well it's happened again! I wrote this article back then, and it applies just as much now. So before we take a look at what games were born this month out of game jams, I want to introduce what game jams are, and why PICO-8 is so good to use in them for anyone new.
What is a Game Jam?
A game jam is an event where you and other developers, artists, designers, and/or musicians come together to create games within a fixed time period, sometimes as long as a month, but more often only spanning from a few hours to a few days. You are challenged to build games based on a given theme or constraints. Game jams encourage experimentation, innovation, and the sharing of knowledge and ideas.
Why PICO-8 is Ideal for Game Jams
PICO-8 stands out as an excellent choice for game jams. Firstly, PICO-8's design philosophy already embraces constraints and these limitations encourage developers to think creatively and limit the scope of their games.
PICO-8 provides a user-friendly environment for rapid prototyping. With its built-in editors for code, sprites, map, SFX and music, developers can swiftly prototype and iterate on their ideas all in one simple program. It's also easy to save, export, and share your project with others, making the logistics of collaboration simple and fast.
The color palette is bright and vibrant while also being able to have high contrast, and this aesthetic helps PICO-8 games stand out from the crowd in a large jam.
Lastly, PICO-8 is accessible to developers of all skill levels. It's lightweight and beginner-friendly. Combine that with comprehensive documentation, and many community-made tutorials ensures a gentle but fast learning curve. The PICO-8 community actively participates in game jams and happily provides valuable support and feedback to each other.

A Game by its Cover Jam 2023
This popular jam, on it's 9th consecutive year since 2015, is probably one of the most relaxed jams and it's thanks to the philosophy of the hosts:
You get an entire month, plus a two week extension in case you feel the stress of the original deadline, and if you want to update your game after that, that's ok too! The rules are simple as well: Find a fictional game cartridge from the My Famicase Exhibit that inspires you, reach out to the artist to get permission, and make a game based on that artist's work.
My Famicase Exhibit
The My Famicase Exhibit, in Tokyo, Japan, is an annual art event organized by the METEOR video game store. The event invites artists from across the globe to design their own unique Famicom cartridge-label. These become physical cartridges displayed in their gallery in Tokyo, and recently also shown in Los Angeles, USA. The exhibit is also always available digitally, and you can browse past years as well.
Jam Participants 2023
There were over 300 participants that initially joined the jam, and of those, 120 final submissions. There were a great variety of game engines used from Unity, Godot, Pyxel, Löve2D, and of course PICO-8! Some were even print-and-play tabletop games. Unfortunately life interviened for quite a few developers who started the jam, but as fate would have it, there were a total of 8 PICO-8 final submissions.
We reached out to these developers to get their perspectives and insights on their game development journeys over the past month and a half so let's hear directly from them about their games!
8 Games By their Covers
(ordered alphabetically)
Apathello
by Josiah Winslow
It was hard to pick just one famicase! There were quite a few that I could've went with, including Kumatori Panic, Peas, and Juicy Blocks, because they looked like they'd translate well to the PICO-8. I chose Apathello mainly because the idea of "outside factors" causing apathy resonated with me, especially at this point in my life.
My concept for the game was that, while you play Othello in this dark void, a mysterious voice from within the void starts talking to you. Just like you, it knows how hard it is to find motivation to do things, what with the state of the world. And it wants to help you through that.
It would be something that would've helped me out greatly if it existed a few years in the past.
Although my last game Ataxxmas had the option for an AI player, its quality left a lot to be desired. This time, I wanted extra care to be put into the AI, to make it the best I could make it. While I learned a lot about various techniques, this ended up taking more time than I thought, and I didn't feel satisfied with the AI until day 32 of the jam!
The last time I released a PICO-8 game was 2020. As for unreleased games, I had been actively working on an online crossword game and a Wordle clone for PICO-8 until the end of 2022. But somewhere along the way, I lost the motivation to complete them.
I don't often participate in game jams, but the fact that AGBIC was going on at a time when I had nothing else to do was a pretty big motivator. It was something to do, and its atmosphere was very chill and unstressful. (The 2-week extension near the end certainly helped calm me down!) I feel like it was the perfect way to get me back into PICO-8 again.
Apathello is a relaxing and atmospheric take on Othello, with 5 levels of AI opponents that are engaging to play against (and to watch play against themselves!). Though I couldn't implement every feature I wanted to, the game is very playable and enjoyable, and I'm happy with it as it is.
Sadly, I wasn't able to fully realize the dialogue feature I envisioned, because I didn't have enough dialogue written in time. But everything in the cart is ready for when I do. And I can't wait to show this feature to the world.
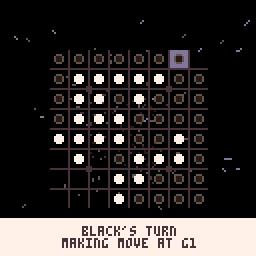
Going Atray
by D3V?
There were many cat themed famicase designs that had peaked my interest, but I finally picked 'Going Astray' as it was really atmospheric. I liked the style of the famicase design, and the idea of cats and souls being tied together really stood out to me.
The original game was supposed to be similar to 'The Witness', in which the main character is supposed to lead souls to their destiny, via following and figuring out rules. However, I made it a metroidvania game because: 1. A puzzle game seemed really cliché for a game with an atmosphere like 'Going Astray', and 2. Because I wanted to dive into the platformer metroidvania genre.
The art was the most difficult part. I wanted a soothing atmosphere to be present, with simple but effective art. However, this ended up taking a lot of sprite space, which was even more limited due to the fact that the lower sprite sheet was taken up by the map. I was reminded that I could use Pico-8's sprite compression system, but I ended up not using it because I considered it as "cheating" because, in my mind, you're supposed to properly manage the limited things of Pico 8, not cheat it. I also found mapping to be a little annoying.
The aesthetics of the game helped motivate me to push through and complete it. Again, I really wanted to nail the atmosphere, so I really wanted to finish the game, to see how it would turn out.
In my opinion, the game turned out alright. I was quite satisfied with the mechanics and such, and I definitely liked the atmosphere - the rain, the cat's sprites, the palette, etc. However, I could've definitely did a better job at fixing a couple of the bugs, and not just go around them. One of them is the infamous collision glitch. Maybe I'll return in the future and fix some of these up, but I'm happy with how it is for now.
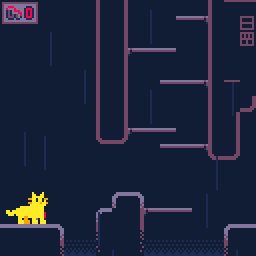
Homunculus
by Nerdy Teachers
This famicase is absolutely gorgeous to me. It is minimal with a lot of white space, emphasizes life with all the greens, and a surprising burst of orange. So it feels quite peaceful but when you notice the strange and intricate protrusions from the head, it makes you stare and wonder. I knew this was the famicase to fuel my imagination and motivation.
I really had no game ideas other than imagining a scientist running through a void white space and searching for sparse plant life to collect. I wanted the game to feel like you are diving in to explore the world of the cover. I made so many decisions along the way that veered away from this original concept completely but I'm very happy with what it became.
The toughest part of developing the game was the initial struggle of deciding what type of game it should be. Thanks to the famicase, I had a clear image of what the game should look like and I started with a mockup of the sprites but I couldn't start coding. I sat for days considering the infinite directions the gameplay could take. It was torture but once the core idea finally struck, it was like the puzzle pieces kept falling into place after that and it was smooth sailing from there.
I had multiple motivations to complete the game. First, the famicase artist's enthusiasm, encouragement, and trust in me made me want to follow through and make him proud. Second, I teach game dev in tiny separate chunks and I wanted to see what I could create by putting them all together in one project. And lastly, I wanted to create a physical famicom cartridge with this game's label to have as a memorable trophy but I needed to earn that by completing the game!
Homunculus is a calm strategic action game with multiple endings that gives you short, medium, and long term goals. The immediate tasks are simple and repetitive so you can learn quickly and then focus on developing your long term strategy to solving the missions. I love playing games that make me strategize and even take notes outside of the game and it makes me so happy to see players doing that with my game!
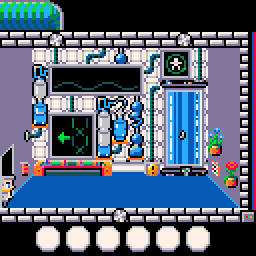
Kumatori Panic!
by Achie
After failing this jam last year by overscoping my project (Occult Nostaliga, sorry RogueCache I'm getting back to that at some point) I was looking for a project that I could create something quick and simple with enough play variation that it is still a good game. Kumatori Panic! was pretty high on that list because the tile matching genre isn't that wild, but also NerdyTeachers just published a Sokoban template I'd love to expand on. The theme also seemed pretty funny, expecially with the expression of the bear.
The original concept was basically a simple sokoban-like pushing mixed with bejeweled-like matching game. Push chicks next to each other, clear them, and progress to the next stage. With this I found I had a cool idea and the general consensus in the Nerdy Lounge and other socials was quite positive, which really kept me going.
The toughest part to be honest was the first day of the release because of a development error. You see, figuring out if you can still complete a level is quite tricky. I had a quick function at first, which became more and more bloated over time. I had 6 different major versions of it and it wasn't working properly in some cases. I just published a new update that will hopefully fix it, but the first day of the release was awful as half of the players couldn't even start due to a bug not letting the game generate levels ....
Pushing through was only possible because the support I got from my fellow developers (shout out to The Quack Pack and the Nerdy Lounge), the enthusiasm from the famicase artist EatSleepMeep, and the support of my fiancé. Without these lovely people I wouldn't have been able to complete the game. You keep these game dev flames from going out in the toughest part of late night debugging.
Kumatori Panic! in the end is in a nice place I feel. EatSleepMeep loved it, fiancé loved it, my close developer friends seemed to enjoy it. It even inspired another creator to make their own sokoban game —what more can you ask for?
The game got a nice visitor viewcount on the first two days of releasing, but only a tiny little fraction of those left behind feedback/comments/shares so I feel in that department it was a bit of a failure. I will try to look into the causes of this (probably the bug I mentioned) and learn from it to better any future games I make.
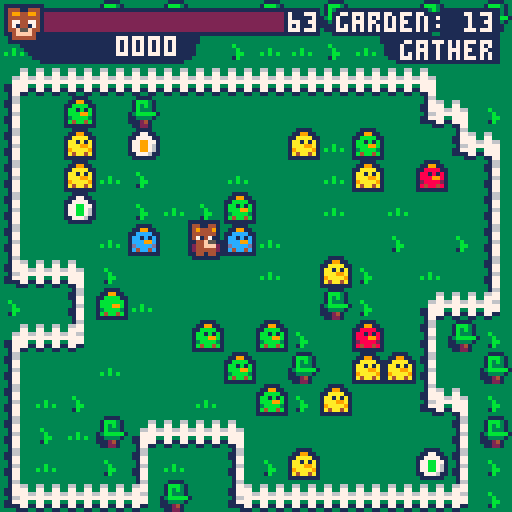
Kumatori Panic!
by PBeS Studios
Hi, this is Gaëtan & Pierre from PBeS Studio, we have been making video games with PICO-8 since 2022. We liked the cute cover art of Kumatori Panic. We very quickly imagined the chicks following the bear in single file, which made us laugh.
Initially, we imagined that we would have to harvest chicks that had escaped from a cage and were wandering in the meadow. Once you picked up the chicks you have to aim and throw them back in the correct cages according to their colors. Then we had the idea of limiting the player movement, taking inspiration from the snake game where you always move straight and just change directions.
Creating the engine was very complex. We had a lot of trouble coding sprites that follow each other. After several tests, we started with the idea that, at each frame, the bear (the player) places an object that includes its direction, its speed and its coordinates in x and y. When a chick collides with this object, it picks up this data and applies it to itself. So we have a line of chicks that make the same movements as the bear with enough time lag to display the entire sprite of the chick. We create the game with what we know and then we adapt to what we are capable of doing.
We found that the feeling of the game was good and that the setting was funny. We wanted to fine tune everything to give a real gaming experience, namely a title screen, a level selection, a tutorial, a presentation of the levels before playing, a victory screen, the animations of the chicks etc. … in short, we really tried to work and make all aspects of the game great.
Our Kumatori Panic! is an arcade game where you have to save chicks from the nugget factory by picking them up in the right order, while avoiding collision. It's quite different from what we imagined at the beginning, but the gaming sensations that we like are still there.
We had positive feedback despite the bugs. We are rather proud and satisfied. We managed to complete the project with a game that we like in all its aspects (code, graphics, music, feelings, etc.). This is also what we defend in video game creation: the idea of going to the end of projects that speak to us and that amuse us while trying out new ways of doing things.
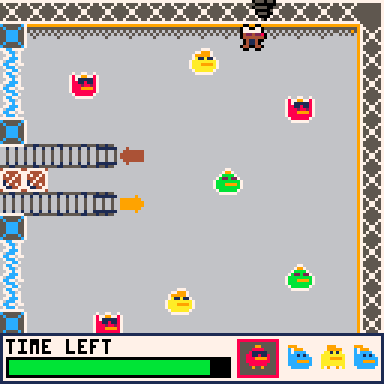
Order Up!
by Alethium
The original art is very low key, and what really stood out to me was the messaging of the artist. “Your task is simple: Deliver the package on time. That's it. No reward for you.” That really struck home as this is something I feel deeply in my bones every day as an artist and creative type among the working class.
I wanted to create an anti-work game that got across the idea that you need to stop and enjoy life in the midst of the grind if you don’t want to succumb to burnout. I developed large portions of Order Up! at work, sitting at my desk, coding among my work duties. If we cannot escape this system, we can at least defy it.
I struggled most with balancing game dev and the other parts of my life. I work full time to pay the rent, which does not leave much time for coding, let alone having a life worth living. When I start a project I have to give myself over to the process fully, or else I will not get enough done fast enough to keep up motivation. I take code breaks after projects to give attention to my partners and my other projects, to make up for this.
It was hectic but I am happy I made it across the finish line. I felt compelled to follow through with the concept because I owed it to myself and my partners to make good on the time I was investing in the project. I couldn't leave my game unfinished; in my mind that meant I would have wasted all the time I poured into it.
Order Up! is a purposefully unfun game with a lot of interesting text hiding in the nooks and crannies. It looks really pretty, too. I was aiming for a play experience that becomes harder than you can deal with, that forces you into a fail state, because work will always ask more of you with no regards to how it’s affecting your health. I wanted to make the things you do to recharge your battery heartfelt and meaningful and I think I succeeded there. Ultimately, I am proud of what I managed to do, and thankful for all the help I got along the way.
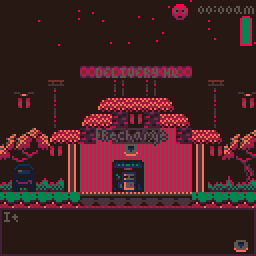
Peas!
by Lucas Castro
I was looking for some Famicase that would refer to a game that was simple to implement, and at the same time interesting. I really wanted to be true to the visuals and description of the Famicase. The peas were circles of the same size, which looked easy to draw. They had different emotions, and there were many of them, which gave me a feeling of comical chaos.
Kevin Gauvin's Famicase description read: "Mix and match the peas in this frenetic puzzle game!". He didn't make it clear what kind of puzzle... initially I thought of a Bubble shooter, but in conversation with my daughter, she brought up the idea of animated Sudoku, which is my mother's favorite puzzle. I loved the idea!! It matched perfectly with the Famicase's proposal and, in addition, it would be a creative possibility to show affection for my dear mother, which also made sense with the more human aspect of this game jam: "embrace the cringe and make art for your loved ones".
It was my first game with Pico-8, my second game jam "taken seriously". There were a few technical challanges but even so, I think the the harder part was to polish the game. In the last two days I shared it with my wife and she brought me a lot of small improvements for the game's progress. As an example, introducing the Pea behaivors with dialogs on the first time it happens and the usage of friendly messages.
My main motivation to complete the game was that I wanted to show the game to my mother (she is 76), and I know that I wouldn't finish it if I had no time pressure that game jams bring. I managed to cut some things and focused on the main game mechanics.
PEAS! Animated Sudoku is the funniest sudoku you have seen. More emotions, nothing of numbers and a pinch of surprises, that pleases even those who don't like the original puzzle. I am super satisfied with the result! Very happy to have given this gift to my mother. Now I am waiting for her to complete the 13th, final level, to see the message I left for her.
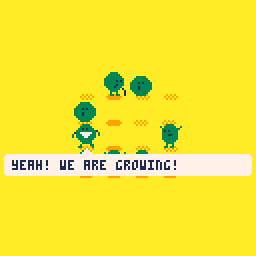
Runaways
by SIC_Benson
The famicase art inspired me, of course. Anton really captured an arcade essence in his cover that spoke to me, but I think the phrase “ENDLESS HIGHWAY CHASE ACTION” really set up shop in my head.
The original game concept was probably pretty similar to how the game turned out --just a motorbike being chased by cop cars in an arcade format. That concept felt achievable despite my lack of coding experience in Pico-8 or in general.
I was following Lazy Dev’s Shmup tutorials and repurposing that for my own concept. So I would get the basics, but then need to adjust it for my own purpose or in a way that wasn’t explicitly laid out in the tutorial. Eventually the base I built on was too basic for the functionality I was going for and I had to rewrite a significant portion of the code. I feel like with the additional mechanics I want to add currently put me in a similar position as well. I think it's just evidence that I’m learning and the code I wrote two weeks or a month ago isn’t as “refined” or “efficient” as I would want moving forward.
I think what kept me motivated was exploring the potential that lies behind the fantasy cartridge. Also, just how relaxed the AGBIC Jam is, knowing that I can continue to work on my entry and make additional updates makes it easier to submit something you might not be 100% happy with.
I hope that “ENDLESS HIGHWAY CHASE ACTION” describes the game well, and if so then I have to be happy with it since that was the goal. I feel like it's a Minimum Viable Product with some definite potential for additional depth and polish with further refinement.
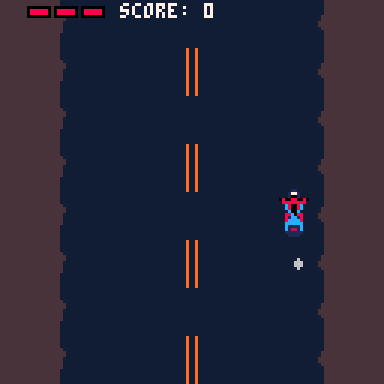

Low Rez Jam
This game jam requires you to develop a game in 64x64 pixel resolution, or less! There are multiple theme options to choose from. This year the theme choices were: Catch ‘Em All; Invasion; Opposite Day; Cooperation; Glitch In The Matrix; Wildfire; Recursive; Creation Story; But Does It Float?; Hidden In Plain Sight.
The jam lasted for 2 weeks, and although there are no rewards, there is a judging system where participants can play and rate each others games which leads to a rankings when its all said and done.
The judging criteria is based on:
(1) Gameplay,
(2) Graphics,
(3) Audio and
(4) Authenticity.
And from those an Overall ranking is given. We have gathered the Low Rez submissions made in PICO-8 and have ordered them here based on their Overall Ranking.
Low Rez Jam
Featured PICO-8 Entries
Ranked out of 304 total submissions!
#7 Outbreak
by Johan Peitz
They say it started in a lab, but no one knows for sure. It doesn't matter anyway, you just need to survive another 10 minutes!
#10 Canyon Crisis
by Jammigans, Fletch, and Mothense
Play as a cowboy amidst an alien invasion! Run through an endless canyon saving cows, shooting aliens, and trying to stay alive in the canyon heat!
#25 Occult Gunner
by Noh
You are an armed robot tasked with defending the wizards bunker. Roll, fly and blast away the invading enemies for as long as you can and fight for a high score!
#41 Sand Trap
by DrSpaceman0
How long can you survive against cascades of falling rainbow sand? Don't let it reach the bottom or its game over!
#47 TetraFabrika
by Extar
Where do all those tetrominoes come from? From the Tetrafabrika, of course! Build tetrominoes as fast as possible in this manic arcade Sokoban-like.
#74 Bitty Dig
by PaloBlanco
Arcade style endless descent as you dig down through tetronimo shaped blocks.
#91 Wooly Ascension
by 4DTri
Escape the farm and rise to the heavens as a sheep.
#101 Bloop! Jump
by Djuniou
You are this small... square? That REALLY REALLY wants to get to the top and get the trophy! Why? We don't know, but they REALLY, REALLY want to get to the top. It wants so bad that everytime it falls, it tries again. And again. AND. AGAIN.
#114 Pyromania
by UltraPotatoDev
Pyromania is about lighting fires in a unspecified forest. This game uses the theme "Wildfire".
#133 HDIEWTG?
by Ari
Full Title: How Do I Even Win This Game?
Join Maya, your favourite comically unhinged raccoon whose only purpose in life is finding out a way to beat this game. Give it your all at these 5 arcade-reminiscent minigames and solve the mystery!
#141 Speedy
by exanimo99
You are a robot dog, sent to find water on mars. You need to juggle charging your battery and avoiding the meteors!!
#199 Hazmat Havoc
by Archonic, VirtuaVirtue & Pico-8 Gamer
Get keycards and unlock doors so you can progress through the facility and get your car keys back, but there's a twist thrown in around halfway through. Keep playing and find out!
#200 Get the Humans 64
by PotatonATopHat
collect as many humans as possible for our experiments.
#218 Pico Draw
by Ray Quaza
A 64x64 drawing application for Pico-8.
Editor's Note: Software tools generally do poorly in game jam ratings, so don't let that turn you away. This is a fun drawing tool with a lot more than first meets the eye.

1-bit Game Jam
Another great jam challenge with a tough restriction is this 1-bit jam, where you can only choose 2 colors to draw in. This year the theme is Light & Dark. This jam is a great way to test your sprite skills by focusing on contrast, not color. As we know, such limitations can really bring out creativity and unique solutions. Despite using only two colors, these games still look amazing.
Featured PICO-8 Entries
Slime's Light
by Werxzy
Slime's light is a puzzle game involving activating/deactivating lamps and doors with light and shadow.
Roast Master
by Liar cool guy
Ever wanted to be a top notch #1 barista worker? Play RoastMaster™ to achieve the highest score without messing up an order!
Maze
by Lucas Castro
You, a flashlight and a lot of care involved in a dark labyrinth. Don't touch the walls, the alarm will go off and you're gone.
Escape from the Lab
by Captain Goodnight
You are RoboX, a friendly robot who was captured by an evil scientist and locked up in his secret lab. Your mission is to escape from the lab and find your way back to your home planet. But be careful! The lab is guarded by fierce robot dogs who will catch you if they see you.
Mothlight
by wabi sabi
The moon is bright, but the nocturnal pollinators are nowhere to be found! Find your moth friends, free them, and pollinate along the way in this short, film noir tale entirely lit by the moon.

Featured Interview
with Jammigans, Fletch, and Mothense
ft. Marina
Marina: So, who are you three and what have you done?
Fletch: Hello! My name is Fletch! I have been making little games for ~2 years now. Mostly just game jams so far. I also stream game development on Twitch and do my best to give it some educational vibes where anyone is welcome to ask questions or make suggestions! This is my first time working with either Jammigans or Mothense but I couldn't be more excited!
Jammigans: ...and I’m Jammigans. Hello! I make pixel art and am trying to get better at making games.
The game we are making is for the LOWREZJAM 2023. The max resolution is 64x64, and one of the themes this year is ”invasion.” We’re using the low-res mode in PICO-8 (64x64) to make a game about a cowboy who will do their best to save the local cows from invading giant insect-like aliens from outer space.
I’m so glad that Fletch wanted to tag along on this jam. It’s such a privilege to have a pro handle the heavy-lifting code so that I can focus mainly on graphics and animations. Speaking of pros, we also have Mothense onboard to contribute with music!
Mothense: Howdy, I'm Mothense, I do music for indie games! Fletch asked me to contribute some music to our jam entry. Him and Jammigans are the driving force behind the project!
Marina: So, how did the three of you find each other? Was it true love at first sight?
Fletch: Jammigans originally reached out to me! A few days into the jam, I reached out to Mothense because we realized we didn't have much hope of composing music ourselves ? I've known both Jammigans and Mothense for a while as mutuals on Twitter! I've admired both of their work for a long time and had them on a hidden "should collab at some point list" but I don't think they knew that. XD
Marina: At what point of the team's development did the idea of "Canyon Crisis" come about?
Jammigans: Me and Fletch spawned ideas on different concepts like the day before the jam started and elaborated on that the first few days of the jam. As the jam started, they also released the optional themes. One of the themes was "invasion".
One of Fletch’s ideas was something like Dodge aliens that descend from the sky by moving to safe squares. As time passes, your points increase, and aliens become increasingly faster. Obstacles like cows might stand in your way or move around the map.
We prioritized the concepts and I did mockups for three of them, one of which was based on that invasion idea. The cows made me think of the wild west. I secretly worked the wild west theme into it the mockup, telling Fletch "I’m twisting the concept a bit, hope that’s ok ? " I also suggested to have the game be about saving the cows instead of them being obstacles. I first suggested the name Chaparral Crisis, thinking about the wild west TV drama ”The High Chaparral”. Fletch suggested swapping ”Chaparral” to ”Canyon”, to make it more recognizable and memorable.
So together with Bunnymaze Dungeon and Wildfire Fighter, Canyon Crisis became our third mockup concept that we polled on social media.
Fletch: I loved your mockups so much. While we were discussing theme ideas, I was doing very basic game infrastructure - creating the foundation of a splash screen, menuing system, tile based movement, sprite sorting, input handling, etc. All of our mockups depended on the same basic systems so I could create the most basics while we took our time really developing a polished concept without wasting time in the jam!
Marina: So, if you could go back and add any feature/thing to the game, what would it be?
Fletch: Better game over experience! The game feels pretty polished given only two weeks of work but the jump cut to game over is jerky and not really smoothed out yet There are also some bugs that players encounter after ~ 20 minutes of play where the terrain disappears thanks to an integer overflow bug that I've fixed and will be released when the jam rating period is over:)
Jammigans: A hundred percent, better game over! I imagine the player character passing out and dropping the hat (maybe slight face reveal?), freeze frame highlighting the cause of the game over. A game-over sound effect and some juicy UI counting up the stats at the end would also be nice.
Other than that, some effects like footstep and slide particle effects, maybe enemies that can take more than one hit, enemy hit/death animation, pieces of wood flying as you destroy barrels, and perhaps dynamite?
Oh, and we got some feedback from a player of the game who suggested a roll move to mix the gameplay up a bit. That could be interesting to experiment with a bit.
Marina: Besides the game over, how did you guys get it so polished in such a short ammount of time?
Jammigans: It helped a lot that we settled quite early on our focus areas. As Fletch did a great job of setting up the gameplay loop and generating the map and pretty much all fundamental programming, I could focus on the visuals, and we asked Mothense to specifically help us out with making the music.
Marina: What was your guy's biggest inspiration for the design of this project?
Jammigans: When I read Fletch’s idea about cows and aliens, I started to imagine a cowboy vs aliens situation. Gun Smoke, Sunset Riders, and Wild Guns are some sources of inspiration for me when it comes to wild west style games. That environment and aesthetic, combined with insect-like monsters, got me started drawing the mockup. Besides that, working with such talented and friendly people inspires me to do my best!
Fletch: Jammigans honestly nailed it as a design lead, I just "yes and" for a few small things but he was the one making decisions about game balance and stuff. Which was great imo because it meant more focus on programming and less on little things that I could fiddle with for hours but would prefer not to. I just made everything a variable and when Jammigans needed it changed I'd go ahead and update the value.
Marina: Well then, what is each of yours' favorite mechanic/feature/ect. in the project?
Fletch: For me, the infinitely scrolling map. I just had a lot of fun puzzling that out haha
Jammigans: I enjoyed how adding just a few sprites and some lerping on top of the movement system made the character come to life. It’s satisfying to see how we can get away with just one single sprite for the running animation, with some offsetting and flipping.
Run sprite:
Movement before lerping and run sprite:
Movement after adding lerping, and the run and slide sprites:
I was also surprised by how just vertically stretching the monster sprite with the SSPR function totally worked as a smear frame for the monster spawn animation. It’s fun to tinker back and forth with code and art for these kind of things.
Monster spawn animation:
Marina: INTERESTING! What was your favorite part about working with each other?
Fletch: I mean, for me it was seeing other people do what I was incapable of doing; having new art or new music be introduced to the project was so fun for me.
Jammigans: Yeah, being able to focus and not having to deal with the anxiety of ”? : yeah you go on tweaking that animation, it’s not like you gonna need any fundamental systems in place to finish this game...”, but instead be assured that the heavy programming and music are taken care of by professionals. Also, the encouragement during the development and bouncing ideas back and forth has been a blessing!
Marina: So, would you consider working together again?
Fletch: Well of course! Mothense and Jammigans are legends!
Jammigans: Absolutely!
Marina: I thank you all for the interview.
- Fletch
- Mothense

Featured Game Review
Fidget Spinner Gone Wrong
by Achie
I’m gonna straight up start with a hot take, I think people are sleeping on this genius, Noh. Every game of theirs is amazing, juiced up, and generally really fun to play! Occult Gunner is no exception, even though the LOWREZJAM put heavy restrictions on the entries (64x64 is tricky) this little gem manages to pack so much into it, that it feels it could burst at any moment.
It starts with introducing the lore of the wizard bunker. Our spellcaster really needs a break to regenerate after the long battles, and after their crow agrees, they initiate the defense robot, our main character! It spins up like crazy and blasts off onto the surface, revealing us the title screen:
Simple, yet effective and foreshadows the high octane gameplay we are gonna get thrown into. But first, controls!
Classic ⬅️➡️ will move you left and right, and ⬇️⬆️ will help you ascend and descend when in what I call, helicopter mode! What, helicopter mode? Yeah, we're gonna bring the choppa to the action! Pressing ❎ will initiate the switch between runner and copter, both serving a different function, while 🅾️ is our dedicated shoot button.
The two different modes serve as two control tools to defend our bunker. Enemies are varied in both land and flying type. Runner mode is fast, but cannot really shoot walking enemies, only up, while flying mode is slower, but can shoot downwards. So the basic logic is to run and gun flying enemies and fly and bomb ground enemies.
Noh thought of this, so there are flying enemies that carry shields either protecting their soft squishy belly or rocking a cool horned helmet to protect from aerial bombing. You can however use the movement speed to shred enemies with your body. Reaching super tempo Terraria movement clouds speeds allow you to hit the next ground enemy you run into.
The goal of the game is really simple. you have three lives to guard, three enemies that can get through you and your endless frantic bulletstorm. After the three lives deplete you will be greeted with a game over scene and a score, so basically the game is a fast paced score chaser!
The juice is so thick you need milkshake straws for it. Bullets have a nice flipped/rotated alternating sprite to help you sell the speed even more, muzzle flash, shockwaves, explosions and just a really frantic gameplay.
The small screen, the offscreen flying enemies and just the fact that you have to run left-right all the time adds so much anxiety of “I need to go here, I need to fly there, ohmygodwhatisdisaladincarpetghost eeee” that it’s a joy to play. The little tinker with the muzzle, the animation (which I think is actually procedural on running mode, if I understood the code correctly, but I’m a dummy) just everything comes together into a nice coherent feel of adrenaline and pumping blood vessels.
Graphically I think I praised it enough, but just look at those enemies. Look at the wizard and the little crow being shocked by the launching drone … it’s just perfect, as every game of theirs. Background is simple enough, but let me tell you, you won’t have the time to look at it. It performs and looks just as good as it needs to be. (Ay, what does that star do over there?)
Sound is crunchy and punchy, bullets feel powerful, explosions crack and the music is a banger track of “Like Clockwork” from Gruber’s amazing Pico-8 Tunes Vol.2 your source of freely available music for PICO-8 projects. It SLAPS like a suburban mom after two mimosas.
In the end this whole experience comes together to an amazing experience, that is going onto my handheld as soon as my battery is charged. Absolutely deserves the #25 placements it achieved out of the 304 entries to jam, if not more! If you are into score chasers or just a really nice 10-15 minutes of adrenaline dose, give it a spin!
“But wait, what about that star?” you might ask, and my answer is, play the game and you will find out! See ya!
About the Author
I stream PICO-8 gameplay and PICO-8 game development on Twitch, as well as write detailed dev logs and a game review series called "Pico Shorts".
Thanks for reading!

PICO-1K Jam 2023
by Liquidream
WHAT is it?
PICO-1K Jam is a fun, relaxed, non-competitive jam which runs for a whole month. Inspired by character-limited jams such as #TweetJam and #TweetTweetJam, where all content (visuals+sound) is generated using just code. The #Pico1k challenges participants to create Games, Demo(scene) and Tools in PICO-8 purely within 1KB of compressed code only - no sprite/map/sound data (unless the submitted code generates it).
WHEN is it?
PICO-1K Jam runs throughout the whole of September. It starts at 7PM (BST) on the 1st, and finishes on the 30th September at the same time.
What are the RULES?
There are only three rules that apply if you wish to submit an entry to PICO-1K Jam (and I really hope you do!) - they are as follows:
-
Your entire entry must be <= 1024 Compressed Bytes in PICO-8 PICO-8 has an easy way to check this (see Jam page for more details)
-
No pre-defined PICO-8 data allowed ALL content must be created using code submitted. You should be able to copy+paste the code into an empty PICO-8 cart & run it.
-
Have fun & be nice… …to yourself, and each other! ?
What can I CREATE in Just 1K?
This is the 3rd year running PICO-1K Jam, and the past two years have had some wonderful entries. Check out the showcases below to see what others managed to create in just 1K…
2021 Entries
When PICO-1K first started (in 2021), the rule limit was based on “Character Count”, but people still managed to create some wonderful entries using just code alone:
2022 Entries
When PICO-8 added the ability to easily view Compressed Bytes, the 2022 jam rules were updated. Moving to a “Compressed Bytes” rule has enabled PICO-1K entries to contain up to double the amount of code than previously, which gives participants more freedom to create some truly wonderful entries:
What are some COMPRESSION tips?
The way that PICO-8 manages its compressed code can seem somewhat like black magic at times (see here for those curious to know how it works! ?).
But here are some tips that may help you on your quest to 1K…
Switch PICO-8 to display “Compressed Bytes” while developing, to ensure you don’t go too far over the limit with your entry that you can’t complete it. (see Jam page for more details)
PICO-8’s compression algorithm seems to work well at pattern-matching chunks of text it has seen before. So you may find that if you have code that repeats similar functionality, making parts of the code exactly the same may compress smaller (rather than assigning a variable and reusing the value) - as the compressor may do it better than you can refactor it.
Use external tools to assist you - such as PXAVIZ, which can show you which parts of your code are consuming the most bytes (and which ones are the most efficient).
Or if you’d rather a more automated solution, try pasting your code into Shrinko8 and let it try to minify it down to as few compressed bytes as it can.
Ultimately, it can be exciting (+infuriating) to get your entry under 1024 Compressed Bytes - but once you achieve it, it can be quite satisfying!
In CLOSING… I hope you can join us for PICO-1K jam 2023 and look forward to seeing what you create. ? If you are able to, don't forget to share your creations (or WIP) using the hashtag #Pico1k ?️?

Cre8: A PICO-8 Jam
by Pico-8 Gamer & Sign
Hi all, Pico-8 Gamer here, and I am one of the new guys in the community with a burning passion to bring PICO-8 to new heights in the game development community. "Why new heights for little ole PICO-8?" some may ask, well it's because along my journey to becoming a game developer, I've been everywhere and tried every game engine.
Now with that, I have gained some knowledge but mostly how to never finish a project. But just when all hope was gone, this little angel called PICO-8 appeared and man, it had everything I ever needed...literally.
Learning PICO-8 has been such a driving force for me, as I've taken time to read the monthly zines as well as enjoy the creations of other developers and being able to pick up something like this is a big milestone for me as I've never really committed to learning code; it's not my strong suit. Despite that, PICO-8 has reignited the passion for game development I obtained back in school while messing with the RPG Maker series and man, is this fire burning strong!
I even made a Physical Console for this lovable thing with some assistance from members of the great community, which lead running into another new developer who has that same fire burning as I do and looking for a way to give back. That person is Sign.
We met within the discord community of PICO-8 and this jam idea sparked and here we are :D.
WHEN is it?
Well to kickstart it off we are doing a lengthy 3 week jam starting on 1st September 2023 until 23rd September! The idea behind this was to give people plenty of time to plan, create, and execute whatever they can think of based on the theme.
What are the RULES?
The rules are pretty simple:
- Your game must be developed using the PICO-8 Fantasy Console
- Follow the theme
- Teams are allowed, so grab your friends or meet new ones to collaborate.
- All assets (sprites, music, sounds) must be original and made during the jam.
What are the REWARDS?
Now for my favorite part, the rewards! This is how we plan to keep this baby full circle and support the developers. The prizes will be Commercially released games and tools made with/for PICO-8! As well as the top 3 of the jams obtaining a secondary prize!
What are the JUDGING CRITERIA?
We wanted to make sure the judging criteria was pretty simple:
- How well does your game follow the theme?
- How fun is your game?
- How well is it presented?
So everything can add points to your score even if you only specialize in one area. An artist, or composer or just a programmer, each area can provide points, follow the theme, boom more points. Just wanted it to be simple.
Who should JOIN this wonderful adventure?
Everyone! New or experienced, everyone has a place here. If you feel like you're uncertain of yourself or your skills, find other developers to team up with, there are plenty of people within the community that match your level of experience. This is all about enjoying the process, the prizes are to support those who wish to take their development career to the next level is all and we support that. So in a way you all joining this jam is also supporting those developers! See, full circle :D!
- Pico-8 Gamer
- Sign

The Death Of A Frog
by Marina
Introduction
I come out of this month from what might be my greatest failure as a game-developer and artist —"Frogs vs Ghosts". This article will cover my mistakes while sharing the lessons I learned from the experience.
Based off art-work by Jake Hall and developed for the "A Game By It's Cover" jam (see above). It took two months to finish near completion, and will continue to be uncompleted.
Although it looked pretty, the game was immensely unfun to play. Which I attribute to two things: OVERSCOPING and LACK OF GAME-CORE.
Overscoping
The worst mistake was made during the most critical part of art, the choosing of which project to pursue. I went over all my options, and chose the one I wanted to make most: "Frogs vs Ghosts". I did not consider the amount of motivation and time it would take to finish my grand turn-based brawler/collect-athon with 16 characters... That's a lie. I assumed I could replenish my motivation, and do more work in less time. This is a text-book case of overscoping.
Motivation in games is like jet-fuel. We chart our trip and hop into our plane. Only half-way to our destination do we realize that we are running low on fuel. Upon realizing the stupidity of our plan, we must now choose a closer destination or worse —crash. The solution to this is to pick a destination we can reach from the get-go. This may seem disheartening, and it is. If we don't want to go somewhere then the motivation will already be low as we begin our trip. My solution? Pick a project you WANT TO MAKE and CAN FINISH.
All potential games fall somewhere on this graph:
Although when you put your project onto this graph you will most likely lie to yourself on the "can finish" axis. One solution to this is to examine the motivation-consumption on your most similar finished project. Another is to peer review your idea and the feasibility of it.
Lack Of Game-Core
The second worst mistake I made during the development of "Frogs vs Ghosts", was not implementing the game-core ASAP. The game-core is the foundational gameplay a game is built on (similar to the core game-loop). "Frogs vs. Ghosts" did not have a game-core until the final days of development where I added in characters with their abilities. Although this could also be defined as a bi-product of poor planning, one thing is a verified result of it: The game was unfun to play due to the fact I was unable to tune-up the game-core.
My justification for this through-out the project was viewing the game as the intended final-product instead of what it was during the moment.
If I Could Go Back
If I could go back in time to the first day of the AGBIC jam; I would pick a few catridges based on how much I liked them, and a few more which I didn't hate but knew they would facilitate easily made games. I would then draw up more in-depth concepts for all of them and see where they lay on the graph above. Finally, I would choose my project by picking the idea with the highest complet-ability and highest motivat-ability.
Conclusion
However, the Delorian is not coming to my rescue. The awful truth is that I wasted two-months and countless hours of sleep on an awful game which was never completed. Do not be like me, ensure that you are planning a game which you can finish.
Thanks & Outtie 5000
Thanks for reading!
If this article is received well I'll consider writing a weekly dev-blog during the month of September where I will: (1) participate in THREE game jams, and (2) make TWO personal projects.
Can I do it? Probably not, but I'm bummed and feel like trying! See you then, and have a good one.

Random Reviews
Game Recommendations on
New Releases: August 2023
Game Jam games weren't the only ones released this month, so as usual, here are the community picked favorites from the general releases that you should play.
Pixel TD
"Omg! Pixel TD is sooooo good!!!!! And for their first game, it's insane! I love it. I can't wait to see more from this developer!"
-Pico-8 Gamer
Tron Legacy
"'The only way to win is to survive.' And the only way to survive is to play, so hop on your lightcycle and fight for the User. Nimbly navigate your way around the mazes, collect familiar looking bonus items, and take on the dangerous Programs that are trying to defrag you. Invite a friend to play and battle for supremacy in the arena, or take on the challenge solo and try to escape the maze."
-KumaKhan22
The Heavens
"The Heavens is an incredibly deep and challenging space sim. You were (probably) born on Earth, but your fate lies in The Heavens!"
-Wolfe3D
Tales of the Arabian Nights
"The jump is old-school and hard to control, but it's that way by deliberate choice! once you accept the awkward jump mechanics, movement itself becomes an interesting puzzle to learn and master. and the variety of stages in this C64 remake, the general polish, and the addition of the stage selector make this a very fun game. Someday I'll come back and clear it all without using the stage select!"
-Pancelor
"An absolutely faithfull remake of the Commodore 64 classic. The levels alternate between single-screen platformer action and side-scrolling shoot-em-up which is old-school, tough as nails gameplay. However Heracluem has added improvements that make the game more accessible. And even if this sort of game is not your thing, you have to try this cart for the incredible music and atmosphere. Very, very highly recommended"
-Street-Monk-1255

Pixel Art Gallery
Artist Spotlight: PJBGamer
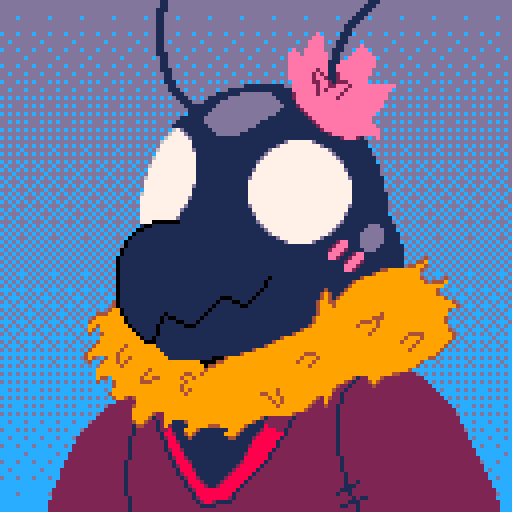
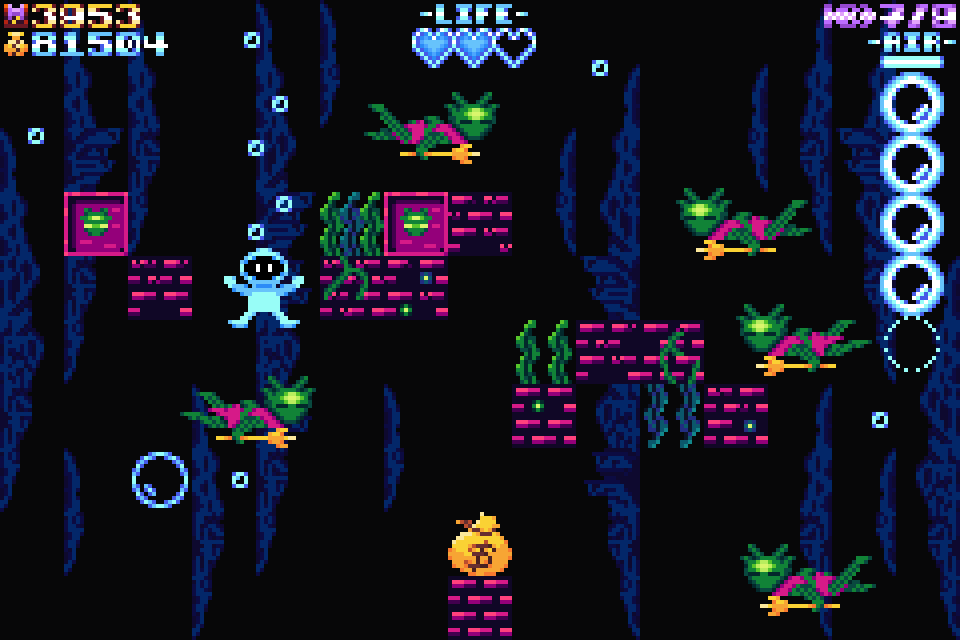
My name's PJBgamer! Although most folks call me either PJ or PJB, either is fine. I'm a pixel artist from the USA trying to learn how to code so that I can make games of my own one day! I'm also trying to get more actively involved in the PICO-8 community since it's a very nice place for up and coming gamedevs. It just feels really nice to share this amazing journey we're all on.

I started learning pixel art around 2018 or so, and I owe a lot of what I learned about pixel art and gamedev to @grogdev on Twitter. If it wasn't for him, I wouldn't even be doing this to the level that I am now. I was also inspired to stream by my friends, which really got me doing art more consistently, and I have them to thank for that as well. One of them is my buddy Wintar, whose character is featured here! BTW Wintar if you're reading this, hi!
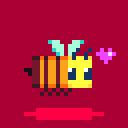
I'd like to leave a message to all PICO-View readers: pursuing your dreams is gonna come with so many challenges. You're gonna be told that you've got your head in the clouds or that you're not taking life seriously. And even some rude people will say that you can't do it. But you shouldn't let those people weigh you down, your dream is important to you, and that's what matters more than anything. And for every one jerk who says you can't, there are 100 awesome folks who say that you can and you will! So don't give up, reach out to the community and I have faith that you can do anything, no matter how difficult it is, because you are never alone in this. After all, you've got to fight to make a wish come true!
Love you all! And I hope you have wonderful lives!
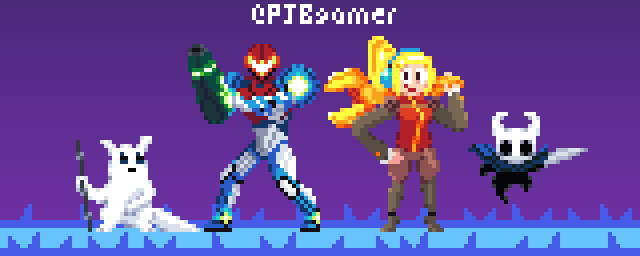

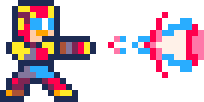
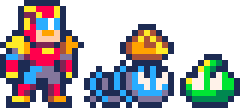
"All of this for a loaf of bread?"

Prototype Party
Each month, this section of Pico-View will bring you a prototype with a focus on a unique mechanic or style. You can take the challenge of playing and getting the highest score or by putting your own spin on this prototype. Feel free to take the code from here and use it however you want!
This month's prototype is named "Color Combo". It is a bare-bones template for building your own game similar to DanceDanceRevolution or Magica.
The main mechanic in this prototype is to detect the combination of button inputs of the arrows in order to create different colors. This game has 6 different colors, which you can turn into elements, potions, weapons, anything! We kept it simple with just color matching.
So there are 3 primary inputs, left, right, and up. And those can be combined into secondary inputs which will create the secondary colors.
left |
blue |
right |
yellow |
up |
red |
left + right |
green |
right + up |
orange |
left + up |
purple |
Play it here:
We challenge you!
Take this prototype as a jump-off point to practice your skills, and add your own flavor to it. The only requirement is to use the mechanic of combining multiple inputs in your game somehow!
How to Share
You can post your spin-off on the BBS, tweet it with the hashtag "#picoview", and join our Discord community if you have questions or want to share your progress. Here is the game cart if you want to see how we made it!

Closing Remarks
Thank you for reading this extra jam filled August issue of the Pico-View web-zine! We hope you enjoyed all of the articles this month. Here are the folks who helped piece the zine together one pixel at a time...
-PJBGamer- Cover Art & Artist Spotlight
-Fletch - Article Writer
-c.diffin - Article Writer
-Liquidream - Article Writer
-Pico-8 Gamer - Article Writer
-Jammigans, Fletch, Mothense - Interviewees
-Marina - Interviewer & Zine-founder
-Achie - Game Reviewer & Article Writer
-Josiah Winslow, D3V?, PBeS Studios, Alethium, Lucas Castro, Sic Benson - Game Jam Interviewees
-Pico-8 Gamer, Wolfe3D, Pancelor, Street-Monk-1255 - Random Reviewers
-NerdyTeachers - Zine-Coordinator, Editor, and Article Writer
Thanks to all the authors, contributors, and readers for supporting our PICO-8 zine! If anyone would like to write an article, share pixel art, or help with anything contact @Marina Makes or @NerdyTeachers on twitter or Discord.
-Nerdy Teachers
10103
29 Aug 2023