FGET
fget
= "flag get"
fget( sprite, [flag] )
sprite | the sprite number of the sprite you want a flag checked. |
flag | (optional) the flag number (0-7) of the flag you want checked. |
This function will compare if the sprite has the flag set or not. If you provide a flag argument then it will return true if that flag is set on that sprite and false if it is not set on that sprite.
If you do not provide a flag argument, then the fget
function will return a single bitfield of all flags that are set on that sprite.
Example:
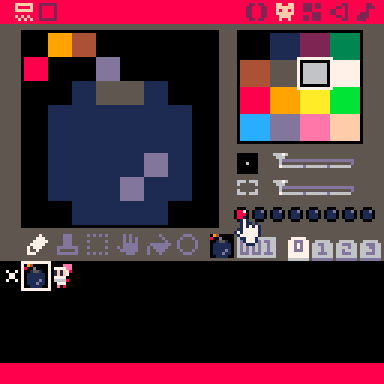
-- bomb= sprite 1, flag 0 on
print( fget(1,0) ) --true
print( fget(1,1) ) --false
Getting Multiple Flags
You can set the flags manually in the sprite editor or with the function fset
, which will do the same. You can have multiple flags turned on for a single sprite. Here we will turn on flag 0 for the bomb sprite and multiple flags on for the man sprite in the sprite editor.
When you hover your mouse over the flags in the sprite editor, the flag number will be displayed at the bottom left of the screen. Notice that flags 1, 3, 5, and 7 are all turned on for the man sprite. So when we ask fget for any of those specific flags it will return true. If we want to know all of the flags that are turned on we don't specify the flag argument in our call for fget, and we need to understand the bitfield number that is returned.
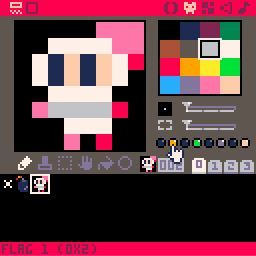
Now let's use this set up to make calls to fget and see what is returned.
-- bomb= sprite 1, flag 0
-- man = sprite 2, flag 1,3,5,7
--bomb
print( fget(1,0) ) --true
print( fget(1,1) ) --false
print( fget(1) ) --1
--man
print( fget(2,0) ) --false
print( fget(2,5) ) --true
print( fget(2) ) --170
Notice that when we specify a flag to be checked, the returning value is either true or false, but when we left out that argument, the bomb sprite returned the number 1
, and the man sprite returned the number 170
. These numbers are bitfields that represent exactly which flags are turned on and they will be a number between 0
(no flags turned on) and 255
(all flags turned on).
To help read these numbers, here is a table to understand which flag or flags are on depending on the bitfield returned.
flag # | bitfield |
---|---|
0 | 1 |
1 | 2 |
2 | 4 |
3 | 8 |
4 | 16 |
5 | 32 |
6 | 64 |
7 | 128 |
By adding the bitfield value of the flags that you want turned on, you will get the unique number that is returned by a sprite that has those specific flags turned on. In our example, the bomb sprite returned the bitfield 1 because only flag 0 was turned on. And the man sprite returned 170
because:
flag #s | bitfields | total |
---|---|---|
1, 3, 5, 7 | 2 + 8 + 32 + 128 | = 170 |
2437
8 Dec 2023